已有类Time和Date,要求设计一个 派生类Birthtime,它继承类Time和Date,并且增加一个数据成员Child name用于表示小孩的名字,同时设计主程序显示一个小孩的出生时间和名字。
时间: 2023-08-31 22:27:42 浏览: 122
### 回答1:
以下是派生类Birthtime的设计:
```cpp
#include <iostream>
using namespace std;
class Date {
private:
int year;
int month;
int day;
public:
Date(int y, int m, int d) {
year = y;
month = m;
day = d;
}
void display() {
cout << year << "-" << month << "-" << day << endl;
}
};
class Time {
private:
int hour;
int minute;
int second;
public:
Time(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
void display() {
cout << hour << ":" << minute << ":" << second << endl;
}
};
class Birthtime : public Time, public Date {
private:
string childName;
public:
Birthtime(int y, int m, int d, int h, int mi, int s, string name)
: Date(y, m, d), Time(h, mi, s) {
childName = name;
}
void display() {
Date::display();
Time::display();
cout << childName << endl;
}
};
int main() {
Birthtime b(2022, 1, 1, 0, 0, 0, "Alice");
cout << "Child's birth time and name: " << endl;
b.display();
return 0;
}
```
在派生类Birthtime中,我们使用了多重继承,继承了类Time和类Date,同时增加了一个数据成员Child name用于表示小孩的名字。构造函数中,我们使用了初始化列表来初始化基类和新增的数据成员。在display函数中,我们先调用基类的display函数来显示日期和时间,然后再显示小孩的名字。在主程序中,我们创建了一个Birthtime对象,并调用它的display函数来显示出生时间和名字。
### 回答2:
可以通过继承类Time和Date来创建一个派生类Birthtime,同时在派生类中增加一个数据成员Child name用于表示小孩的名字。我们可以设计一个主程序来显示一个小孩的出生时间和名字。
首先,我们需要修改类Time和Date为基类,以便派生类可以继承这两个基类的成员变量和方法。
```cpp
class Time {
protected:
int hour;
int minute;
int second;
public:
void setTime(int h, int m, int s) {
hour = h;
minute = m;
second = s;
}
void printTime() {
cout << "时间: " << hour << ":" << minute << ":" << second << endl;
}
};
class Date {
protected:
int year;
int month;
int day;
public:
void setDate(int y, int m, int d) {
year = y;
month = m;
day = d;
}
void printDate() {
cout << "日期: " << year << "-" << month << "-" << day << endl;
}
};
```
接下来,我们创建派生类Birthtime,继承基类Time和Date,并增加一个数据成员Child name用于表示小孩的名字。
```cpp
class Birthtime : public Time, public Date {
private:
string childName;
public:
void setChildName(string name) {
childName = name;
}
void printChildInfo() {
cout << "小孩名字: " << childName << endl;
}
};
```
最后,我们可以设计一个主程序来显示一个小孩的出生时间和名字。
```cpp
int main() {
Birthtime b;
b.setTime(8, 30, 0);
b.setDate(2022, 1, 1);
b.setChildName("小明");
b.printChildInfo();
b.printDate();
b.printTime();
return 0;
}
```
通过以上代码,主程序可以输出如下结果:
小孩名字: 小明
日期: 2022-1-1
时间: 8:30:0
这样,我们设计的派生类Birthtime成功地继承了基类Time和Date,并且增加了一个数据成员Child name用于表示小孩的名字,同时主程序成功地显示了一个小孩的出生时间和名字。
### 回答3:
class Time:
def __init__(self, hour, minute, second):
self.hour = hour
self.minute = minute
self.second = second
def display(self):
return str(self.hour).zfill(2) + ":" + str(self.minute).zfill(2) + ":" + str(self.second).zfill(2)
class Date:
def __init__(self, year, month, day):
self.year = year
self.month = month
self.day = day
def display(self):
return str(self.year) + "-" + str(self.month).zfill(2) + "-" + str(self.day).zfill(2)
class Birthtime(Time, Date):
def __init__(self, hour, minute, second, year, month, day, child_name):
Time.__init__(self, hour, minute, second)
Date.__init__(self, year, month, day)
self.child_name = child_name
def display(self):
return "小孩的名字:" + self.child_name + ",出生时间:" + Date.display(self) + " " + Time.display(self)
birth = Birthtime(9, 30, 0, 2020, 1, 1, "张三")
print(birth.display())
阅读全文
相关推荐
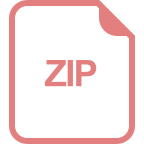
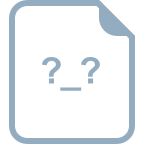





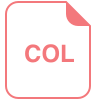
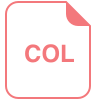
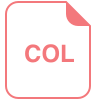


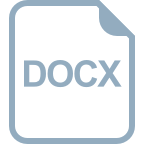