C++中const全局常量与const函数内常量的区别
时间: 2024-03-17 16:41:38 浏览: 16
C++中的const关键字可以用来定义常量,它的作用是使变量的值不可修改。但是在不同的使用场景下,const关键字的含义可能会有所不同。
全局常量是在程序开头定义的,它的作用域是从定义处到文件结尾,可以被整个文件内的函数访问。定义全局常量时,需要在前面加上const关键字,表示这是一个常量。例如:
```
const int MAX = 100; // 定义全局常量MAX
```
函数内常量是在函数内部定义的,只能在该函数内部使用。定义函数内常量时,同样需要在前面加上const关键字,表示这是一个常量。例如:
```
void func()
{
const int MAX = 100; // 定义函数内常量MAX
}
```
区别在于,全局常量的作用域是整个文件,而函数内常量的作用域仅限于该函数。此外,全局常量在程序运行期间都是存在于内存中的,而函数内常量只在函数执行期间存在于内存中,在函数执行完毕后就被销毁了。
相关问题
const 函数 是成员函数 还是全局函数
const 是 C++ 中的一个关键字,用来修饰数据类型、指针、函数等,表示它们是常量。在这里,const 并不是函数的类型,因此不能说 const 函数是成员函数还是全局函数。
在 C++ 中,const 成员函数是一种特殊的成员函数,它被声明为 const 类型,表示该函数不会修改对象的状态。const 成员函数可以被 const 对象调用,也可以被非 const 对象调用,但是非 const 对象调用 const 成员函数时,只能调用该函数的 const 版本。例如:
```c++
class Example {
public:
void func() const {
// const 成员函数
}
void func() {
// 非 const 成员函数
}
};
int main() {
const Example ex1;
ex1.func(); // 只能调用 const 版本的 func
Example ex2;
ex2.func(); // 调用非 const 版本的 func
const Example& ex3 = ex2;
ex3.func(); // 只能调用 const 版本的 func
return 0;
}
```
在上面的例子中,我们定义了一个类 Example,其中包含两个名为 func 的函数,一个是 const 成员函数,一个是非 const 成员函数。在 main 函数中,我们分别定义了三个对象 ex1、ex2 和 ex3,其中 ex1 是 const 对象,ex2 是非 const 对象,ex3 是 ex2 的一个 const 引用。
当我们调用 ex1.func 时,只能调用 const 版本的 func;而当我们调用 ex2.func 时,只能调用非 const 版本的 func;当我们调用 ex3.func 时,也只能调用 const 版本的 func。
C++怎么定义常量,常量存放在内存的哪个位置,const修饰成员函数的目的是什么
在C++中,可以使用关键字`const`来定义常量。常量的值不可以被修改,可以用来表示程序中不可变的数据。常量可以在函数内部、类内部或全局范围内定义。
在函数内部定义常量时,可以使用以下语法: `const type name = value;`
在类内部定义常量时,可以使用以下语法:`static const type name = value;`
在全局范围内定义常量时,可以使用以下语法:`#define name value` 或 `const type name = value;`
常量存放在内存的位置取决于它们的类型和作用域。对于局部常量,它们存储在堆栈中。对于全局常量,它们存储在静态存储区中。
`const`修饰成员函数的目的是为了防止该函数修改对象的状态。当成员函数被声明为`const`时,它不能修改对象的成员变量,也不能调用非`const`的成员函数。这样做有助于确保对象的状态不会被意外改变,并提高程序的安全性。
相关推荐
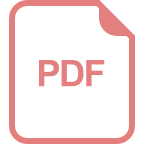
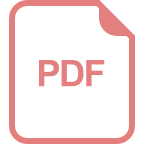
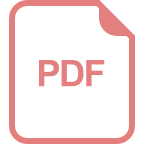












