现需要编写一个简易快递计价程序。具体来说: 1、抽象快递类Express,其包含一个属性int weight表示快递重量(单位为kg),一个方法getWeight()用于返回快递重量和一个抽象方法getTotal()用于计算快递运费。 2、两个类继承Express,分别是: (a)顺路快递SLExpress:计价规则为首重(1kg)12元,每增加1kg费用加2元。 (b)地地快递DDExpress:计价规则为首重(1kg)5元,每增加1kg费用加1元。 3、Main: 接收用户通过控制台输入的N行信息,自动计算所有快递的运费总和。
时间: 2024-03-05 10:55:13 浏览: 64
下面是实现题目要求的完整代码:
```java
import java.util.Scanner;
// 抽象快递类 Express
abstract class Express {
int weight; // 快递重量(单位:kg)
Express(int weight) {
this.weight = weight;
}
public int getWeight() {
return weight;
}
public abstract int getTotal(); // 计算快递运费
}
// 顺路快递类 SLEXpress
class SLEXpress extends Express {
SLEXpress(int weight) {
super(weight);
}
public int getTotal() {
int baseFee = 12; // 首重费用
int extraFee = 2; // 超重每kg费用
if (weight <= 1) {
return baseFee;
} else {
return baseFee + extraFee * (weight - 1);
}
}
}
// 地地快递类 DDExpress
class DDExpress extends Express {
DDExpress(int weight) {
super(weight);
}
public int getTotal() {
int baseFee = 5; // 首重费用
int extraFee = 1; // 超重每kg费用
if (weight <= 1) {
return baseFee;
} else {
return baseFee + extraFee * (weight - 1);
}
}
}
// 测试类 Main
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int totalFee = 0; // 快递总运费
while (scanner.hasNext()) {
String expressType = scanner.next();
int weight = scanner.nextInt();
Express express;
if (expressType.equals("SL")) {
express = new SLEXpress(weight);
} else if (expressType.equals("DD")) {
express = new DDExpress(weight);
} else {
continue; // 如果输入的快递类型不是 SL 或 DD,则跳过本次循环
}
totalFee += express.getTotal(); // 计算快递总运费
}
scanner.close();
System.out.println(totalFee);
}
}
```
程序首先定义了一个抽象类 Express,包含了一个 weight 属性和一个 getTotal() 抽象方法,用于计算快递运费。然后分别定义了 SLEXpress 和 DDExpress 两个类,分别继承了 Express 类,并实现了 getTotal() 方法。这两个类的实现分别对应了题目中的计价规则。
最后在 Main 类中,使用 Scanner 读入多组数据,判断输入的快递类型是 SL 还是 DD,再根据输入的快递重量创建相应的对象,调用 getTotal() 方法计算快递运费并累加到总运费中,最后输出总运费。如果输入的快递类型不是 SL 或 DD,则跳过本次循环。
阅读全文
相关推荐
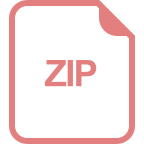




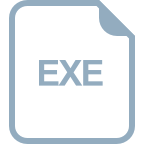
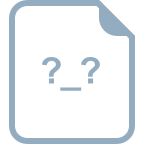
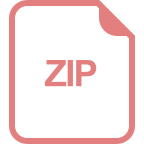
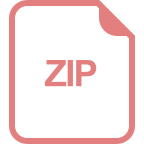
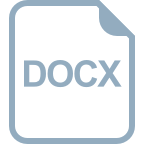
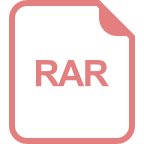
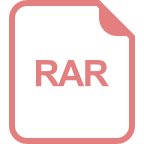
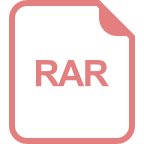
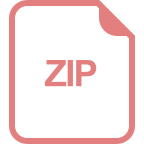
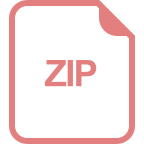