首页使用ASE读取POSCAR,提取所有C原子的坐标,计算C原子在晶胞中的平均位置和平均分布范围。要求1:具体代码,越简单越好;要求2.能识别出POSCAR中的化学元素C原子,避免出现找不到C原子的情况
使用ASE读取POSCAR,提取所有C原子的坐标,计算C原子在晶胞中的平均位置和平均分布范围。要求1:具体代码,越简单越好;要求2.能识别出POSCAR中的化学元素C原子,避免出现找不到C原子的情况
时间: 2024-03-19 10:45:54 浏览: 131
以下是Python代码实现,使用pymatgen库来读取POSCAR文件和分析晶体结构:
```python
from pymatgen.core.structure import Structure
# 读取POSCAR文件
structure = Structure.from_file('POSCAR')
# 获取所有C原子的坐标
c_positions = []
for i, site in enumerate(structure):
if site.specie.symbol == 'C':
c_positions.append(site.coords)
# 计算C原子在晶胞中的平均位置和平均分布范围
c_positions = np.array(c_positions)
c_mean = np.mean(c_positions, axis=0)
c_std = np.std(c_positions, axis=0)
print("C原子在晶胞中的平均位置为:", c_mean)
print("C原子在晶胞中的平均分布范围为:", c_std)
```
以上代码首先使用`from_file`方法读取POSCAR文件,然后遍历晶体结构中的每一个原子,如果其元素符号为C,则将其坐标添加到列表`c_positions`中。最后,计算`c_positions`数组的均值和标准差,即得到C原子在晶胞中的平均位置和平均分布范围。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
大家在看

《数据库原理与应用》大作业.zip
数据库,酒店点菜管理系统

基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目,含有代码注释,新手也可看懂,个人手打98分项目,导师非常认可的高分项目,毕业设计、期末大作业和课程设计高分必看,下载下来,简单部署,就可以使用。
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目
基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目基于时空图卷积(ST-GCN)的骨骼动作识别(python源码+项目说明)高分项目基于时空图卷积(ST

基于Matlab绘制风向与风速的关系图.zip.zip
1.版本:matlab2014/2019a,内含运行结果,不会运行可私信
2.领域:智能优化算法、神经网络预测、信号处理、元胞自动机、图像处理、路径规划、无人机等多种领域的Matlab仿真,更多内容可点击博主头像
3.内容:标题所示,对于介绍可点击主页搜索博客
4.适合人群:本科,硕士等教研学习使用
5.博客介绍:热爱科研的Matlab仿真开发者,修心和技术同步精进,matlab项目合作可si信

关于初始参数异常时的参数号-无线通信系统arm嵌入式开发实例精讲
5.1 接通电源时的故障诊断
接通数控系统电源时,如果数控系统未正常启动,发生异常时,可能是因为驱动单元未
正常启动。请确认驱动单元的 LED 显示,根据本节内容进行处理。
LED显示 现 象 发生原因 调查项目 处 理
驱动单元的轴编号设定
有误
是否有其他驱动单元设定了
相同的轴号
正确设定。
NC 设定有误 NC 的控制轴数不符 正确设定。
插头(CN1A、CN1B)是否
已连接。
正确连接
AA 与 NC 的初始通信未正常
结束。
与 NC 间的通信异常
电缆是否断线 更换电缆
设定了未使用轴或不可
使用。
DIP 开关是否已正确设定 正确设定。
插头(CN1A、CN1B)是否
已连接。
正确连接
Ab 未执行与 NC 的初始通
信。
与 NC 间的通信异常
电缆是否断线 更换电缆
确认重现性 更换单元。12 通过接通电源时的自我诊
断,检测出单元内的存储
器或 IC 存在异常。
CPU 周边电路异常
检查驱动器周围环境等是否
存在异常。
改善周围环
境
如下图所示,驱动单元上方的 LED 显示如果变为紧急停止(E7)的警告显示,表示已
正常启动。
图 5-3 NC 接通电源时正常的驱动器 LED 显示(第 1 轴的情况)
5.2 关于初始参数异常时的参数号
发生初始参数异常(报警37)时,NC 的诊断画面中,报警和超出设定范围设定的异常
参数号按如下方式显示。
S02 初始参数异常 ○○○○ □
○○○○:异常参数号
□ :轴名称
在伺服驱动单元(MDS-D/DH –V1/V2)中,显示大于伺服参数号的异常编号时,由于
多个参数相互关联发生异常,请按下表内容正确设定参数。
87

微电子实验器件课件21
1. 肖特基势垒二极管工艺流程及器件结构
2. 编写该器件的 Athena 程序,以得到器件精确的结构图
3. 定义初始衬底
5. 沉积 Pt 薄膜并剥离
6.
最新推荐

034-基于AT89C52的矩阵键盘扫描proteus仿真设计.rar
51单片机

双级式储能模型,可做充放电转以及低电压故障穿越,含有负序抑制模块,可做对称故障与不对称故障
双级式储能模型,可做充放电转以及低电压故障穿越,含有负序抑制模块,可做对称故障与不对称故障

郑州升达大学2024-2025第一学期计算机视觉课程期末试卷,
郑州升达大学2024-2025第一学期计算机视觉课程期末试卷,原版。配套教材为《OpenCV计算机视觉基础教程》夏帮贵主编。

金工实习线上考试线切割课后试题.docx
线切割课后试题

网络原理课程设计【校园网规划】+思科模拟器,包含pkt文件及完整实验报告,附录含有源码
目录 摘 要 1 一、设计任务概述 3 1.1 设计目的 3 1.2 项目任务和要求 3 1.3 参考资料 3 二、项目开发环境 4 三、项目需求分析 5 四、 项目设计和实现 5 4.1 总体设计 5 4.2 功能设计 6 4.3 系统实现 7 五、系统运行和测试 12 六、设计总结 15 七、附录 16 7.1 程序清单 16 7.2 其他需要说明的内容 23。内容来源于网络分享,如有侵权请联系我删除。另外如果没有积分的同学需要下载,请私信我。

探索zinoucha-master中的0101000101奥秘
资源摘要信息:"zinoucha:101000101"
根据提供的文件信息,我们可以推断出以下几个知识点:
1. 文件标题 "zinoucha:101000101" 中的 "zinoucha" 可能是某种特定内容的标识符或是某个项目的名称。"101000101" 则可能是该项目或内容的特定代码、版本号、序列号或其他重要标识。鉴于标题的特殊性,"zinoucha" 可能是一个与数字序列相关联的术语或项目代号。
2. 描述中提供的 "日诺扎 101000101" 可能是标题的注释或者补充说明。"日诺扎" 的含义并不清晰,可能是人名、地名、特殊术语或是一种加密/编码信息。然而,由于描述与标题几乎一致,这可能表明 "日诺扎" 和 "101000101" 是紧密相关联的。如果 "日诺扎" 是一个密码或者编码,那么 "101000101" 可能是其二进制编码形式或经过某种特定算法转换的结果。
3. 标签部分为空,意味着没有提供额外的分类或关键词信息,这使得我们无法通过标签来获取更多关于该文件或项目的信息。
4. 文件名称列表中只有一个文件名 "zinoucha-master"。从这个文件名我们可以推测出一些信息。首先,它表明了这个项目或文件属于一个更大的项目体系。在软件开发中,通常会将主分支或主线版本命名为 "master"。所以,"zinoucha-master" 可能指的是这个项目或文件的主版本或主分支。此外,由于文件名中同样包含了 "zinoucha",这进一步确认了 "zinoucha" 对该项目的重要性。
结合以上信息,我们可以构建以下几个可能的假设场景:
- 假设 "zinoucha" 是一个项目名称,那么 "101000101" 可能是该项目的某种特定标识,例如版本号或代码。"zinoucha-master" 作为主分支,意味着它包含了项目的最稳定版本,或者是开发的主干代码。
- 假设 "101000101" 是某种加密或编码,"zinoucha" 和 "日诺扎" 都可能是对其进行解码或解密的钥匙。在这种情况下,"zinoucha-master" 可能包含了用于解码或解密的主算法或主程序。
- 假设 "zinoucha" 和 "101000101" 代表了某种特定的数据格式或标准。"zinoucha-master" 作为文件名,可能意味着这是遵循该标准或格式的最核心文件或参考实现。
由于文件信息非常有限,我们无法确定具体的领域或背景。"zinoucha" 和 "日诺扎" 可能是任意领域的术语,而 "101000101" 作为二进制编码,可能在通信、加密、数据存储等多种IT应用场景中出现。为了获得更精确的知识点,我们需要更多的上下文信息和具体的领域知识。

【Qt与OpenGL集成】:提升框选功能图形性能,OpenGL的高效应用案例
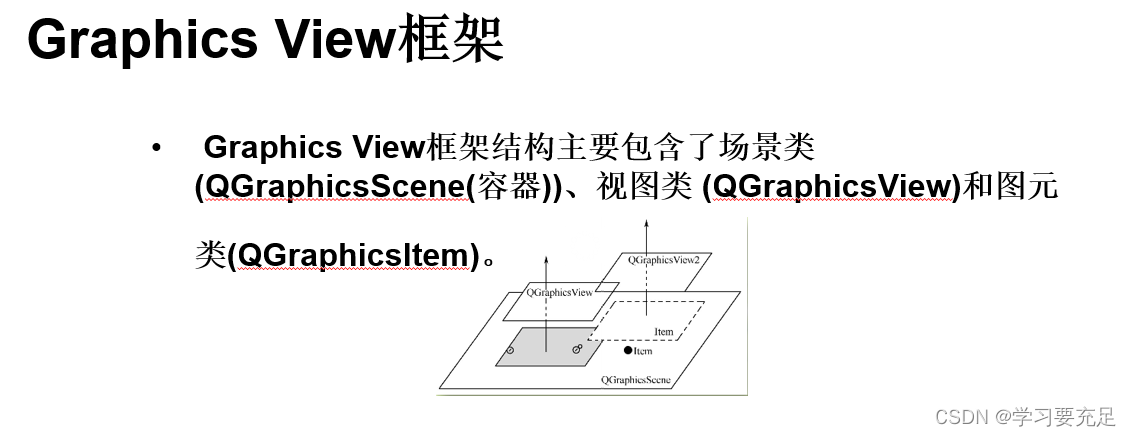
# 摘要
本文旨在探讨Qt与OpenGL集成的实现细节及其在图形性能优化方面的重要性。文章首先介绍了Qt与OpenGL集成的基础知识,然后深入探讨了在Qt环境中实现OpenGL高效渲染的技术,如优化渲染管线、图形数据处理和渲染性能提升策略。接着,文章着重分析了框选功能的图形性能优化,包括图形学原理、高效算法实现以及交互设计。第四章通过高级案例分析,比较了不同的框选技术,并探讨了构

ffmpeg 指定屏幕输出
ffmpeg 是一个强大的多媒体处理工具,可以用来处理视频、音频和字幕等。要使用 ffmpeg 指定屏幕输出,可以使用以下命令:
```sh
ffmpeg -f x11grab -s <width>x<height> -r <fps> -i :<display>.<screen>+<x_offset>,<y_offset> output_file
```
其中:
- `-f x11grab` 指定使用 X11 屏幕抓取输入。
- `-s <width>x<height>` 指定抓取屏幕的分辨率,例如 `1920x1080`。
- `-r <fps>` 指定帧率,例如 `25`。
- `-i

个人网站技术深度解析:Haskell构建、黑暗主题、并行化等
资源摘要信息:"个人网站构建与开发"
### 网站构建与部署工具
1. **Nix-shell**
- Nix-shell 是 Nix 包管理器的一个功能,允许用户在一个隔离的环境中安装和运行特定版本的软件。这在需要特定库版本或者不同开发环境的场景下非常有用。
- 使用示例:`nix-shell --attr env release.nix` 指定了一个 Nix 环境配置文件 `release.nix`,从而启动一个专门的 shell 环境来构建项目。
2. **Nix-env**
- Nix-env 是 Nix 包管理器中的一个命令,用于环境管理和软件包安装。它可以用来安装、更新、删除和切换软件包的环境。
- 使用示例:`nix-env -if release.nix` 表示根据 `release.nix` 文件中定义的环境和依赖,安装或更新环境。
3. **Haskell**
- Haskell 是一种纯函数式编程语言,以其强大的类型系统和懒惰求值机制而著称。它支持高级抽象,并且广泛应用于领域如研究、教育和金融行业。
- 标签信息表明该项目可能使用了 Haskell 语言进行开发。
### 网站功能与技术实现
1. **黑暗主题(Dark Theme)**
- 黑暗主题是一种界面设计,使用较暗的颜色作为背景,以减少对用户眼睛的压力,特别在夜间或低光环境下使用。
- 实现黑暗主题通常涉及CSS中深色背景和浅色文字的设计。
2. **使用openCV生成缩略图**
- openCV 是一个开源的计算机视觉和机器学习软件库,它提供了许多常用的图像处理功能。
- 使用 openCV 可以更快地生成缩略图,通过调用库中的图像处理功能,比如缩放和颜色转换。
3. **通用提要生成(Syndication Feed)**
- 通用提要是 RSS、Atom 等格式的集合,用于发布网站内容更新,以便用户可以通过订阅的方式获取最新动态。
- 实现提要生成通常需要根据网站内容的更新来动态生成相应的 XML 文件。
4. **IndieWeb 互动**
- IndieWeb 是一个鼓励人们使用自己的个人网站来发布内容,而不是使用第三方平台的运动。
- 网络提及(Webmentions)是 IndieWeb 的一部分,它允许网站之间相互提及,类似于社交媒体中的评论和提及功能。
5. **垃圾箱包装/网格系统**
- 垃圾箱包装可能指的是一个用于暂存草稿或未发布内容的功能,类似于垃圾箱回收站。
- 网格系统是一种布局方式,常用于网页设计中,以更灵活的方式组织内容。
6. **画廊/相册/媒体类型/布局**
- 这些关键词可能指向网站上的图片展示功能,包括但不限于相册、网络杂志、不同的媒体展示类型和布局设计。
7. **标签/类别/搜索引擎**
- 这表明网站具有内容分类功能,用户可以通过标签和类别来筛选内容,并且可能内置了简易的搜索引擎来帮助用户快速找到相关内容。
8. **并行化(Parallelization)**
- 并行化在网站开发中通常涉及将任务分散到多个处理单元或线程中执行,以提高效率和性能。
- 这可能意味着网站的某些功能被设计成可以同时处理多个请求,比如后台任务、数据处理等。
9. **草稿版本+实时服务器**
- 草稿版本功能允许用户保存草稿并能在需要时编辑和发布。
- 实时服务器可能是指网站采用了实时数据同步的技术,如 WebSockets,使用户能够看到内容的实时更新。
### 总结
上述信息展示了一个人在个人网站开发过程中所涉及到的技术和功能实现,包括了环境配置、主题设计、内容管理和用户体验优化。从使用Nix-shell进行环境隔离和依赖管理到实现一个具有高级功能和良好用户体验的个人网站,每个技术点都是现代Web开发中的关键组成部分。

Qt框选功能的国际化实践:支持多语言界面的核心技术解析

# 摘要
本文系统地探讨了Qt框架下多语言界面设计与国际化的实现原理和技术细节。首先介绍了Qt国际化框架的基础知识和多语言界面设计的基本原理,包括文本处理、资源文件管理、核心API的应用等。随后,文章详细阐述了设计可翻译用户界面、动态语言切换和界面更新以及测试和调试多语言界面的实践技巧。深入理解