// Draw spiral inside the triangle using VBO glBindBuffer(GL_ARRAY_BUFFER, spiralVBO); glEnableClientState(GL_VERTEX_ARRAY); glVertexPointer(3, GL_FLOAT, 0, nullptr); glDrawArrays(GL_LINE_STRIP, 0, spiralVertices.size() / 3); glDisableClientState(GL_VERTEX_ARRAY);
时间: 2023-12-30 07:05:15 浏览: 182
这段代码的作用是使用VBO在三角形内部绘制螺旋线。首先,通过函数glBindBuffer将VBO绑定到OpenGL的顶点数组缓冲区上。然后,启用顶点数组并设置指针,告诉OpenGL如何解释缓冲区中的数据。接下来,使用glDrawArrays函数绘制线段,其中GL_LINE_STRIP表示绘制一系列连接的线段,顶点数量为spiralVertices.size() / 3。最后,禁用顶点数组并解绑VBO。
相关问题
x = self.x_origin + (j + 0.5) * self.pixel_width y = self.y_origin + (i + 0.5) * self.pixel_height z = val self.iteSize += 1 return x, y, z dsm_path = 'C:\sanwei\jianmo\Productions\Production_2\Production_2_DSM_part_2_2.tif' dsm_data = gdal.Open(dsm_path) dsm_array = dsm_data.ReadAsArray() spiral_iterator = SpiralIterator(dsm_array,x=810,y=500) while spiral_iterator.hasNext(): x, y, z = spiral_iterator.get() print(f'Value at ({x},{y}):{z}')这段代码怎么改可以将打印的坐标保存为文档
你可以将打印出来的坐标以及对应的值写入到一个文本文件中,方法和上面类似。以下是修改后的代码示例:
```python
import gdal
class SpiralIterator:
def __init__(self, array, x=0, y=0):
self.array = array
self.x_origin = x
self.y_origin = y
self.pixel_width = 1
self.pixel_height = 1
self.iteSize = 0
self.i = 0
self.j = 0
self.di = 0
self.dj = 1
self.width = array.shape[1]
self.height = array.shape[0]
def hasNext(self):
return self.iteSize < self.width * self.height
def get(self):
val = self.array[self.i, self.j]
x = self.x_origin + (self.j + 0.5) * self.pixel_width
y = self.y_origin + (self.i + 0.5) * self.pixel_height
self.iteSize += 1
self.i, self.j, self.di, self.dj = self.getNextIndex()
return x, y, val
def getNextIndex(self):
if (self.i + self.di < 0 or self.i + self.di == self.height
or self.j + self.dj < 0 or self.j + self.dj == self.width
or self.array[self.i + self.di, self.j + self.dj] is None):
self.di, self.dj = self.dj, -self.di
return self.i + self.di, self.j + self.dj, self.di, self.dj
dsm_path = r'C:\sanwei\jianmo\Productions\Production_2\Production_2_DSM_part_2_2.tif'
dsm_data = gdal.Open(dsm_path)
dsm_array = dsm_data.ReadAsArray()
spiral_iterator = SpiralIterator(dsm_array, x=810, y=500)
# 打开文件
with open('coordinates.txt', 'w') as f:
while spiral_iterator.hasNext():
x, y, z = spiral_iterator.get()
print(f'Value at ({x},{y}): {z}')
# 将坐标和对应的值写入文件
f.write(f'({x},{y}): {z}\n')
print('Done!')
```
在这个例子中,我们使用了Python的with语句来打开文件,它可以自动管理文件的打开和关闭,避免了手动关闭文件时可能出现的错误。在while循环中,我们将打印的坐标和对应的值写入到了文件中。文件的名字可以根据你的需要自行修改。运行结束后,你可以在相应的目录下找到保存好的coordinates.txt文件。
def extract_building_shadow(image, dsm, ground_spacing, radius): shadow_mask = np.zeros_like(image, dtype=np.bool) for i in range(0, image.shape[0], ground_spacing): for j in range(0, image.shape[1], ground_spacing): if not np.any(shadow_mask[i, j]): center = (i, j) ground_height = dsm[i, j] for x, y in spiral_search(center, radius, ground_spacing): if x < 0 or x >= image.shape[0] or y < 0 or y >= image.shape[1]: continue if np.any(shadow_mask[x, y:]): continue height = dsm[x, y] if height > ground_height: shadow_mask[x, y] = True elif height == ground_height: if np.linalg.norm(np.array([x, y]) - np.array(center)) < \ np.linalg.norm(np.array([i, j]) - np.array(center)): shadow_mask[x, y] = True return shadow_mask image = cv2.imread('C:\yingxiang\DJI_20230322140516_0026_V.JPG') dsm_path = 'C:/sanwei/jianmo/Productions/Production_2/Production_2_DSM_part_2_2.tif' dsm_dataset = gdal.Open(dsm_path) output_path = 'C:\yingxiang\shadow_mask.png'这段代码怎么改可以将掩膜结果保存
您可以在函数返回结果时添加以下代码将掩膜结果保存为 PNG 文件:
```
cv2.imwrite(output_path, shadow_mask.astype(np.uint8) * 255)
```
这将把 `shadow_mask` 数组中的布尔值转换为 0 和 1 的整数,并将其乘以 255 然后转换为无符号 8 位整数,最后使用 OpenCV 的 `imwrite()` 函数将其保存为 PNG 文件。请确保 `output_path` 变量包含正确的文件路径和文件名。
阅读全文
相关推荐
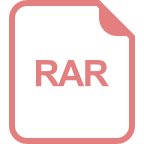
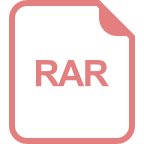
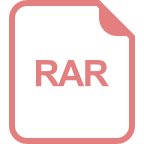
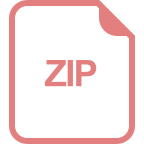
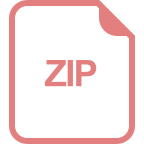
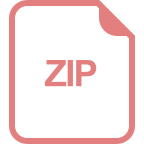
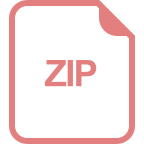
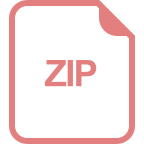
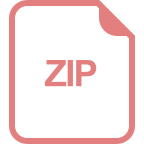
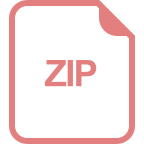
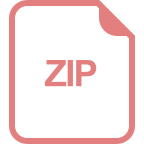


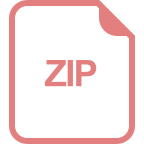
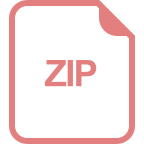