c语言编写程序,先讲字符串s中的字符按正序存放到t串中,然后把s中的字符按逆序连接到t串的后面
时间: 2024-05-07 21:21:15 浏览: 28
下面是C语言的程序实现:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char s[100], t[200];
int len, i, j;
printf("请输入字符串s:");
gets(s);
len = strlen(s);
for(i = 0; i < len; i++)
{
t[i] = s[i]; // 把s中的字符按正序存放到t串中
}
for(j = 0; j < len; j++)
{
t[i+j] = s[len-j-1]; // 把s中的字符按逆序连接到t串的后面
}
t[i+j] = '\0'; // 在t串的末尾加上'\0',形成一个字符串
printf("连接后的字符串t为:%s\n", t);
return 0;
}
```
运行程序后,输入一个字符串s,程序就会把s中的字符按正序存放到t串中,然后把s中的字符按逆序连接到t串的后面,最后输出连接后的字符串t。
相关问题
设计一个C语言程序,先将在字符串s中的字符按正序存放到t串中,然后把s中的字符按逆序连接到t串的后面
好的,下面是我为你编写的程序,注意:程序只能处理字符数组,不能处理字符串。
```c
#include <stdio.h>
#include <string.h>
void reverse(char *str, int len) {
int i, j;
char temp;
for (i = 0, j = len - 1; i < j; i++, j--) {
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
}
void reverse_concatenate(char *s, int len, char *t) {
int i, j;
for (i = 0; i < len; i++) {
t[i] = s[i];
}
reverse(s, len);
for (j = 0; j < len; j++) {
t[i + j] = s[j];
}
}
int main() {
char s[] = "hello world";
int len = strlen(s);
char t[len * 2];
reverse_concatenate(s, len, t);
printf("s = %s\nt = %s\n", s, t);
return 0;
}
```
程序的思路是,先将s中的字符正序存放到t中,然后将s中的字符反转,并连接到t的后面。reverse函数用于反转字符串,reverse_concatenate函数用于完成题目要求。在程序中,假设s字符串长度为len,t字符串长度为len*2(因为t需要存储s和s的反转)。
用c语言写一个功能:先将在字符串s中的字符按正序存放到t串中 字符按逆序连接到t串的后面。
以下是用 C 语言实现将字符串 s 中的字符按正序存放到 t 串中,字符按逆序连接到 t 串的后面的代码:
```c
#include <stdio.h>
#include <string.h>
void reverse(char *s, char *t) {
int i, j, len;
len = strlen(s);
// 将 s 中的字符按正序存放到 t 中
for (i = 0, j = 0; i < len; i++, j++) {
t[j] = s[i];
}
// 将 s 中的字符按逆序连接到 t 的后面
for (i = len - 1, j = len; i >= 0; i--, j++) {
t[j] = s[i];
}
// 在 t 的末尾添加字符串结束符 '\0'
t[len * 2] = '\0';
}
int main() {
char s[100], t[200];
printf("Please enter the string s: ");
scanf("%s", s);
reverse(s, t);
printf("The result is: %s\n", t);
return 0;
}
```
这个函数接受两个参数,分别为字符串 s 和 t,其中 s 为传入的参数,t 为函数内部生成的字符串。函数先将 s 中的字符按正序存放到 t 中,然后再将 s 中的字符按逆序连接到 t 的后面,最后在 t 的末尾添加字符串结束符 '\0'。主函数中调用了这个函数,并输出结果。
相关推荐
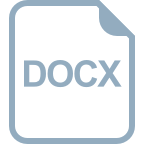












