java这一段批量下载图片代码
时间: 2023-08-31 08:33:23 浏览: 70
### 回答1:
以下是使用Java批量下载图片的代码示例:
```java
import java.io.*;
import java.net.URL;
public class BatchImageDownloader {
public static void main(String[] args) throws Exception {
String imageUrl = "https://example.com/image.jpg"; // 替换为你要下载的图片URL
String destinationFolder = "C:/images/"; // 替换为你要保存图片的文件夹路径
// 创建保存图片的文件夹
File folder = new File(destinationFolder);
if (!folder.exists()) {
folder.mkdir();
}
// 下载图片
for (int i = 1; i <= 10; i++) { // 下载10张图片,可根据需求修改
URL url = new URL(imageUrl);
String fileName = "image" + i + ".jpg"; // 每张图片的文件名
File file = new File(destinationFolder + fileName);
InputStream is = url.openStream();
OutputStream os = new FileOutputStream(file);
byte[] b = new byte[2048];
int length;
while ((length = is.read(b)) != -1) {
os.write(b, 0, length);
}
is.close();
os.close();
System.out.println("下载完成:" + fileName);
}
}
}
```
该代码可以将一个指定URL的图片下载到指定的文件夹中,并自动命名为`image1.jpg`、`image2.jpg`、`image3.jpg`,以此类推。你可以根据自己的需要修改代码中的图片URL、保存文件夹路径以及下载图片的数量。
### 回答2:
批量下载图片可以使用Java的IO流和网络编程功能实现。以下是一个简单的示例代码:
```java
import java.io.*;
import java.net.URL;
public class ImageDownloader {
public static void main(String[] args) {
String[] imageUrls = {"https://example.com/image1.jpg", "https://example.com/image2.jpg"};
for (String imageUrl : imageUrls) {
try {
URL url = new URL(imageUrl);
InputStream inputStream = url.openStream();
String fileName = imageUrl.substring(imageUrl.lastIndexOf("/") + 1);
FileOutputStream outputStream = new FileOutputStream(fileName);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
inputStream.close();
outputStream.close();
System.out.println("图片下载成功:" + fileName);
} catch (IOException e) {
e.printStackTrace();
System.out.println("图片下载失败:" + imageUrl);
}
}
}
}
```
以上代码中,将需要下载的图片URL存储在一个字符串数组中。然后使用循环遍历数组,依次下载每个图片。
首先,通过URL类创建一个URL对象,并打开其输入流。然后,从URL的输入流中读取数据,并将数据写入本地文件的输出流。循环读取和写入,直到没有数据可读。
最后,关闭输入流和输出流,并打印下载成功的信息。
需要注意的是,示例代码中的图片URL是一个示例,请替换为真实的图片URL。同时,在处理网络请求和文件IO时,应该使用try-catch语句来捕获可能发生的异常,以便及时处理并避免程序异常退出。
### 回答3:
以下是一段用Java编写的批量下载图片的代码:
```java
import java.io.*;
import java.net.URL;
import java.util.ArrayList;
import java.util.List;
public class ImageDownloader {
public static void main(String[] args) {
// 图片链接列表
List<String> imageUrls = new ArrayList<>();
imageUrls.add("http://example.com/image1.jpg");
imageUrls.add("http://example.com/image2.jpg");
imageUrls.add("http://example.com/image3.jpg");
// 图片保存路径
String savePath = "C:/images/";
// 创建保存路径
File directory = new File(savePath);
if (!directory.exists()) {
directory.mkdirs();
}
// 下载图片
for (String imageUrl : imageUrls) {
try {
// 构建URL对象
URL url = new URL(imageUrl);
// 打开链接
InputStream inputStream = url.openStream();
// 构建保存路径
String fileName = imageUrl.substring(imageUrl.lastIndexOf("/") + 1);
String fileSavePath = savePath + fileName;
// 创建输出流
OutputStream outputStream = new FileOutputStream(fileSavePath);
// 缓冲区大小
byte[] buffer = new byte[1024];
// 从输入流读取数据,并写入输出流
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
// 关闭输入流和输出流
inputStream.close();
outputStream.close();
System.out.println("成功下载图片:" + fileName);
} catch (IOException e) {
System.out.println("下载图片出错:" + e.getMessage());
}
}
}
}
```
以上代码通过URL对象打开链接,然后通过输入流将图片数据读取到缓冲区,并通过输出流将缓冲区中的数据写入指定的保存路径。通过循环遍历图片链接列表,可以实现批量下载图片的功能。在代码中,需要将imageUrls列表替换为实际的图片链接列表,savePath替换为保存路径即可。
相关推荐
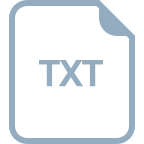
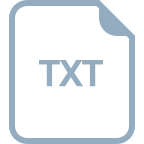














