实现KMP算法,C++
时间: 2023-07-11 10:04:43 浏览: 120
以下是C++实现的KMP算法:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
void getNext(string s, vector<int>& next) {
int i = 0, j = -1;
next[0] = -1;
while(i < s.length() - 1) {
if(j == -1 || s[i] == s[j]) {
i++;
j++;
next[i] = j;
}
else {
j = next[j];
}
}
}
int kmp(string s1, string s2) {
vector<int> next(s2.length(), -1);
getNext(s2, next);
int i = 0, j = 0;
while(i < s1.length() && j < s2.length()) {
if(j == -1 || s1[i] == s2[j]) {
i++;
j++;
}
else {
j = next[j];
}
}
if(j == s2.length()) {
return i - j;
}
return -1;
}
int main() {
string s1 = "hello world";
string s2 = "world";
int pos = kmp(s1, s2);
if(pos != -1) {
cout << "匹配成功,位置为:" << pos << endl;
}
else {
cout << "匹配失败" << endl;
}
return 0;
}
```
其中,`getNext`函数用于生成模式串`s2`的`next`数组,`kmp`函数用于在文本串`s1`中查找模式串`s2`。若查找成功,返回匹配的位置;否则返回-1。
阅读全文
相关推荐



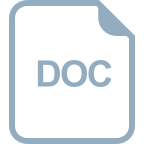










