c语言写滑动电子相册在触摸屏上代码
时间: 2024-02-23 12:59:24 浏览: 73
以下是一个简单的C语言代码示例,用于在触摸屏上创建滑动电子相册:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <linux/input.h>
#include <pthread.h>
#include <gtk/gtk.h>
#define MAX_IMAGE_COUNT 10
int fd;
pthread_t tid;
char *image_files[MAX_IMAGE_COUNT];
int current_image_index = 0;
void load_image_files() {
int count = 0;
DIR *dir;
struct dirent *ent;
if ((dir = opendir ("photos")) != NULL) {
while ((ent = readdir (dir)) != NULL) {
if (count >= MAX_IMAGE_COUNT) break;
if (strstr(ent->d_name, ".jpg") || strstr(ent->d_name, ".png")) {
char *filename = malloc(strlen(ent->d_name) + 1);
strcpy(filename, ent->d_name);
image_files[count++] = filename;
}
}
closedir (dir);
}
}
void *input_thread(void *arg) {
struct input_event ev;
while (1) {
read(fd, &ev, sizeof(struct input_event));
if (ev.type == EV_KEY && ev.code == BTN_TOUCH && ev.value == 1) {
current_image_index++;
if (current_image_index >= MAX_IMAGE_COUNT) {
current_image_index = 0;
}
gtk_main_iteration_do(FALSE);
}
}
}
void show_current_image() {
GtkWidget *image = gtk_image_new_from_file(image_files[current_image_index]);
gtk_widget_show(image);
}
int main(int argc, char *argv[]) {
load_image_files();
GtkWidget *window = gtk_window_new(GTK_WINDOW_TOPLEVEL);
gtk_window_set_title(GTK_WINDOW(window), "Photo Album");
gtk_window_set_position(GTK_WINDOW(window), GTK_WIN_POS_CENTER);
gtk_container_set_border_width(GTK_CONTAINER(window), 10);
GtkWidget *image = gtk_image_new();
gtk_container_add(GTK_CONTAINER(window), image);
show_current_image();
gtk_widget_show_all(window);
fd = open("/dev/input/event0", O_RDONLY);
pthread_create(&tid, NULL, input_thread, NULL);
gtk_main();
close(fd);
return 0;
}
```
这个示例程序将从名为“photos”的文件夹中加载所有的“.jpg”和“.png”文件,并在触摸屏上显示它们。用户可以通过轻触屏幕来滑动浏览相册中的不同照片。这个示例程序使用了GTK+图形用户界面工具包来创建窗口和图像视图,并使用了Linux输入事件API来检测触摸屏输入。
阅读全文
相关推荐
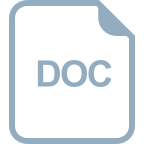






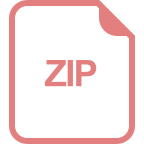
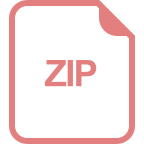
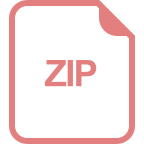
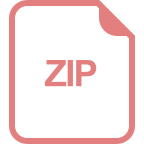
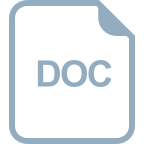
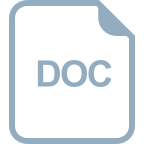