Delphi 创建个数组 往里面添加数字 并判断数字是否存在
时间: 2024-11-17 10:29:40 浏览: 1
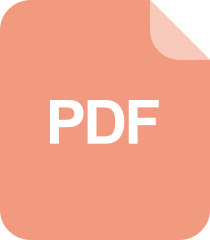
利用for循环把遍历数组中的元素依次获取出来 数组笔记及基本知识点.pdf
在Delphi中创建一个数组并在其中添加数字并判断其是否存在的过程如下:
首先,我们需要定义一个整数类型的数组,例如,假设我们创建一个包含0-9的数组:
```delphi
const MAX_VALUE = 10;
type
NumbersArray = array[0..MAX_VALUE] of Integer;
var
arrNumbers: NumbersArray;
```
然后我们可以创建这个数组并初始化:
```delphi
arrNumbers := [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
```
如果你想向数组中添加新的数字,比如添加一个新数值`NewNum`,可以用`SetLength`函数扩展现有数组,或者直接索引访问并赋值:
```delphi
SetLength(arrNumbers, Length(arrNumbers) + 1); // 扩展数组
arrNumbers[arrNumbers.Length - 1] := NewNum; // 或者直接赋值
```
接下来,我们可以通过循环遍历数组,使用`InStr`或`Pos`函数来判断一个特定的数字是否存在:
```delphi
function ContainsNumber(const NumberToFind: Integer): Boolean;
var
Index: Integer;
begin
Index := Low(arrNumbers);
while Index <= High(arrNumbers) do
begin
if arrNumbers[Index] = NumberToFind then
Result := True;
Exit; // 数字找到了,结束搜索
Inc(Index);
end;
Result := False; // 如果没找到,则返回False
end;
```
现在你可以调用`ContainsNumber`函数来检查某个数字是否在数组中:
```delphi
if ContainsNumber(5) then
WriteLn('5 exists in the array.');
else
WriteLn('5 does not exist in the array.');
```
阅读全文
相关推荐
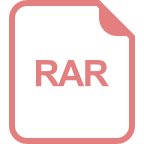
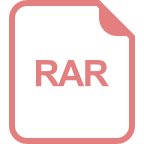
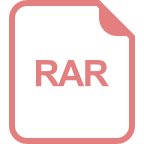
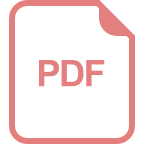
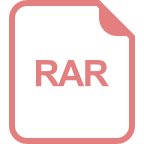
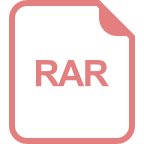
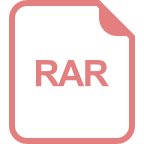
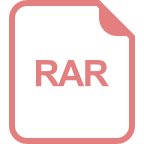
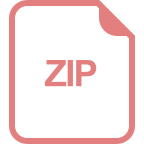
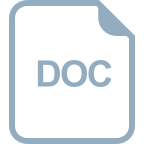
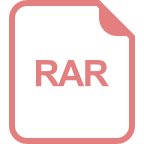
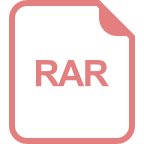
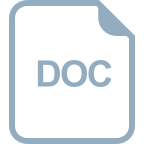
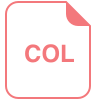