实现c#导入excel数据并与数据库对比
时间: 2023-12-18 18:02:07 浏览: 80
可以使用C#中的ExcelDataReader库来读取Excel文件中的数据,然后使用ADO.NET连接数据库,将Excel数据与数据库中的数据进行比较。以下是一个简单的示例代码:
```csharp
using System.Data;
using System.Data.OleDb;
using ExcelDataReader;
// 定义Excel文件路径和连接字符串
string filePath = @"C:\example.xlsx";
string connectionString = "Provider=Microsoft.ACE.OLEDB.12.0;Data Source=" + filePath + ";Extended Properties=\"Excel 12.0;HDR=YES;IMEX=1\"";
// 使用ExcelDataReader读取Excel文件
using (var stream = File.Open(filePath, FileMode.Open, FileAccess.Read))
{
using (var reader = ExcelReaderFactory.CreateReader(stream))
{
// 将Excel数据存储在DataTable中
var excelData = new DataSet();
excelData.Tables.Add(reader.AsDataSet().Tables[0]);
// 使用ADO.NET连接数据库
using (var connection = new OleDbConnection(connectionString))
{
connection.Open();
// 从数据库中获取数据,存储在DataTable中
var dbData = new DataTable();
var command = new OleDbCommand("SELECT * FROM tablename", connection);
var adapter = new OleDbDataAdapter(command);
adapter.Fill(dbData);
// 比较Excel数据和数据库数据
foreach (DataRow excelRow in excelData.Tables[0].Rows)
{
foreach (DataRow dbRow in dbData.Rows)
{
if (excelRow[0].ToString() == dbRow[0].ToString() && excelRow[1].ToString() == dbRow[1].ToString())
{
// 如果数据一致,则不做任何处理
break;
}
else
{
// 如果数据不一致,则进行相应处理,例如更新数据库中的数据
}
}
}
}
}
}
```
需要注意的是,这里使用的是OleDb连接字符串,需要安装Microsoft Access Database Engine才能正常使用。另外,如果Excel文件中包含多个工作表,需要通过reader.AsDataSet().Tables[index]来指定需要读取的工作表。
阅读全文
相关推荐
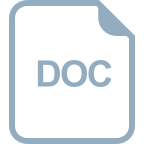
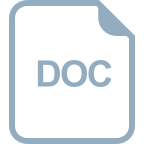
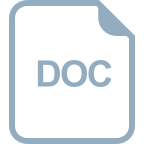
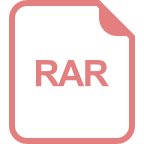
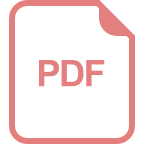
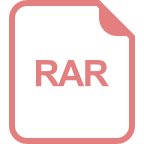
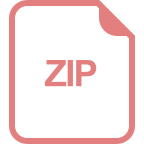
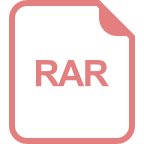
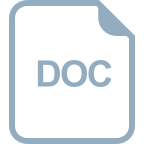
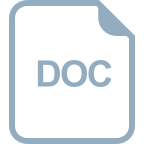
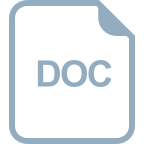
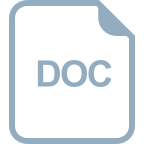
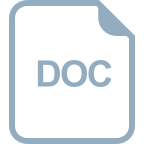
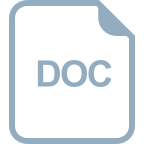
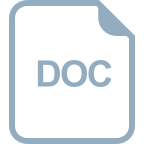

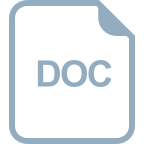
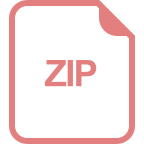
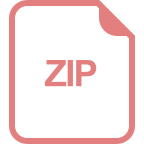