智能车左右扫线然后拟合曲线并且找到拐点python
时间: 2023-11-19 09:44:07 浏览: 102
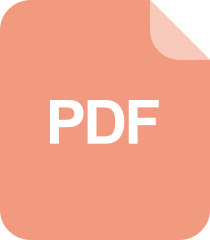
面向复杂路网低频GPS采样数据新型地图匹配算法
智能车左右扫线可以通过摄像头获取图像,然后使用图像处理技术进行处理。具体的步骤如下:
1. 获取图像:使用摄像头获取智能车前方的图像。
2. 图像预处理:对图像进行预处理,比如灰度化、二值化、滤波等操作,以方便后续的图像处理。
3. 边缘检测:使用边缘检测算法(如Sobel算子、Canny算子等)检测图像中的边缘。
4. 直线拟合:根据边缘检测的结果,使用直线拟合算法(如Hough变换)拟合出左右两条直线。
5. 曲线拟合:将左右两条直线进行曲线拟合,得到左右两条曲线。
6. 拐点检测:在左右两条曲线中检测拐点,拐点即为转弯点。
以下是一个简单的 Python 代码示例,用于实现上述步骤中的左右扫线并拟合曲线:
```
import cv2
import numpy as np
# 设置ROI区域
roi = [(0, 200), (640, 200), (640, 480), (0, 480)]
# 设置阈值
threshold = 100
# 获取摄像头
cap = cv2.VideoCapture(0)
while True:
# 读取图像
ret, frame = cap.read()
# 转换为灰度图像
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 设置ROI区域
mask = np.zeros_like(gray)
roi_corners = np.array([roi], dtype=np.int32)
cv2.fillPoly(mask, roi_corners, 255)
masked_gray = cv2.bitwise_and(gray, mask)
# 边缘检测
edges = cv2.Canny(masked_gray, 50, 150, apertureSize=3)
# 左右扫线
left_line = []
right_line = []
for i in range(200, 480):
left_found = False
right_found = False
for j in range(0, 320):
if edges[i, j] > threshold:
left_line.append((j, i))
left_found = True
break
for j in range(639, 320, -1):
if edges[i, j] > threshold:
right_line.append((j, i))
right_found = True
break
if not left_found:
left_line.append((0, i))
if not right_found:
right_line.append((639, i))
# 曲线拟合
left_fit = np.polyfit([p[1] for p in left_line], [p[0] for p in left_line], 2)
right_fit = np.polyfit([p[1] for p in right_line], [p[0] for p in right_line], 2)
# 拐点检测
left_derivative = np.polyder(left_fit)
right_derivative = np.polyder(right_fit)
left_root = np.roots(left_derivative)
right_root = np.roots(right_derivative)
left_turn_point = (int(left_root[0]), int(np.polyval(left_fit, left_root[0])))
right_turn_point = (int(right_root[0]), int(np.polyval(right_fit, right_root[0])))
# 显示结果
result = cv2.cvtColor(edges, cv2.COLOR_GRAY2BGR)
for p in left_line:
cv2.circle(result, p, 2, (0, 0, 255), -1)
for p in right_line:
cv2.circle(result, p, 2, (0, 255, 0), -1)
cv2.line(result, (int(left_fit[0] * 480 ** 2 + left_fit[1] * 480 + left_fit[2]), 480), (int(left_fit[0] * 200 ** 2 + left_fit[1] * 200 + left_fit[2]), 200), (0, 0, 255), 2)
cv2.line(result, (int(right_fit[0] * 480 ** 2 + right_fit[1] * 480 + right_fit[2]), 480), (int(right_fit[0] * 200 ** 2 + right_fit[1] * 200 + right_fit[2]), 200), (0, 255, 0), 2)
cv2.circle(result, left_turn_point, 5, (0, 0, 255), -1)
cv2.circle(result, right_turn_point, 5, (0, 255, 0), -1)
cv2.imshow('result', result)
# 按下ESC键退出程序
if cv2.waitKey(1) == 27:
break
# 释放摄像头
cap.release()
# 关闭窗口
cv2.destroyAllWindows()
```
注意:这只是一个简单的示例,实际应用中需要根据具体情况进行调整和优化。
阅读全文
相关推荐
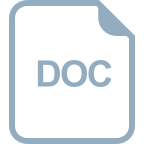
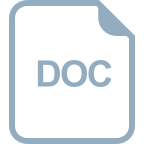
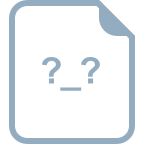
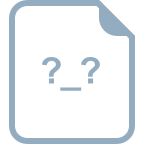
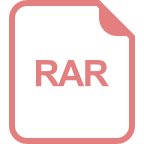
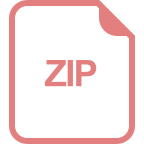
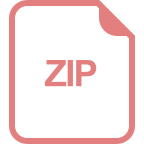
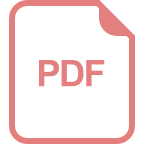
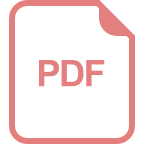
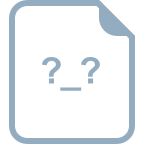
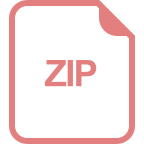
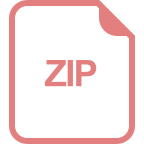
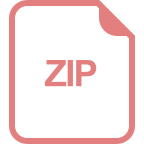
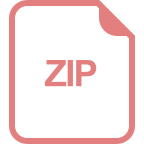
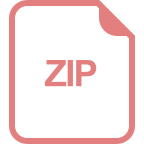
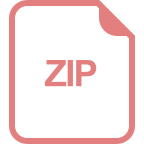
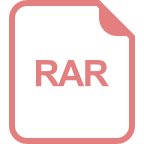
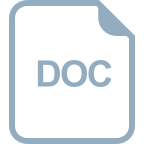