用java编写一个银行家算法,有如下要求:1创建二维数组等数据结构用来表示进程对资源的使用情况,相关数据包括进程名称、进程数目、资源数目、最大需求数目、已分配数目、仍需数目及当前状态下可利用数目等。 2.在用户输入所有初始化信息后,首先对信息以矩阵形式输出,再输入一组可用资源数目,此时系统判断是否存在安全序列,若有则输出一个安全序列;若无,则表示初始化不正确,请重新输入。 3.在已经处于安全状态的前提下,某进程提出新的资源申情,包括进程名称、申请资源数目等,先对用户提出的请求进行合法性检查,若请求合法,则进行试分配。最后对试分配后的状态,调用安全性检查算法进行是否存在安全序列的判断。若安全,给出分配后资源使用情况,并输出一个安全序列。否则,拒绝申请并打印出相应的拒绝原因。
时间: 2024-03-12 12:45:03 浏览: 73
下面是一个Java实现的银行家算法,满足您的所有要求:
```java
import java.util.Scanner;
public class BankersAlgorithm {
// 进程类
class Process {
String name; // 进程名称
int[] maxClaim; // 最大需求数目
int[] allocated; // 已分配数目
int[] need; // 仍需数目
boolean finished; // 是否完成
boolean aborted; // 是否中止
Process(String name, int[] maxClaim, int[] allocated) {
this.name = name;
this.maxClaim = maxClaim;
this.allocated = allocated;
this.need = new int[maxClaim.length];
for (int i = 0; i < maxClaim.length; i++) {
need[i] = maxClaim[i] - allocated[i];
}
this.finished = false;
this.aborted = false;
}
void print() {
System.out.printf("%-10s", name);
for (int i = 0; i < maxClaim.length; i++) {
System.out.printf("%-10d", maxClaim[i]);
}
for (int i = 0; i < allocated.length; i++) {
System.out.printf("%-10d", allocated[i]);
}
for (int i = 0; i < need.length; i++) {
System.out.printf("%-10d", need[i]);
}
System.out.println();
}
boolean canFinish(int[] available) {
for (int i = 0; i < need.length; i++) {
if (need[i] > available[i]) {
return false;
}
}
return true;
}
void allocate(int[] request) {
for (int i = 0; i < allocated.length; i++) {
allocated[i] += request[i];
need[i] -= request[i];
}
}
void release(int[] request) {
for (int i = 0; i < allocated.length; i++) {
allocated[i] -= request[i];
need[i] += request[i];
}
}
}
// 资源类
class Resource {
String name; // 资源名称
int[] available; // 当前状态下可利用数目
Resource(String name, int[] available) {
this.name = name;
this.available = available;
}
}
// 全局变量
int n; // 进程数目
int m; // 资源数目
Process[] processes; // 进程数组
Resource resource; // 资源对象
// 初始化函数
void init() {
Scanner scanner = new Scanner(System.in);
// 输入进程数目和资源数目
System.out.print("Enter the number of processes: ");
n = scanner.nextInt();
System.out.print("Enter the number of resources: ");
m = scanner.nextInt();
// 创建进程数组和资源对象
processes = new Process[n];
for (int i = 0; i < n; i++) {
System.out.print("Enter the name of process " + i + ": ");
String name = scanner.next();
System.out.print("Enter the max claim of process " + i + ": ");
int[] maxClaim = new int[m];
for (int j = 0; j < m; j++) {
maxClaim[j] = scanner.nextInt();
}
System.out.print("Enter the allocated resources of process " + i + ": ");
int[] allocated = new int[m];
for (int j = 0; j < m; j++) {
allocated[j] = scanner.nextInt();
}
processes[i] = new Process(name, maxClaim, allocated);
}
System.out.println("Process\tMax Claim\tAllocated\tNeed");
for (int i = 0; i < n; i++) {
processes[i].print();
}
// 创建资源对象
System.out.print("Enter the available resources: ");
int[] available = new int[m];
for (int i = 0; i < m; i++) {
available[i] = scanner.nextInt();
}
resource = new Resource("resource", available);
}
// 安全性检查
boolean isSafe() {
int[] work = resource.available.clone();
boolean[] finish = new boolean[n];
for (int i = 0; i < n; i++) {
finish[i] = false;
}
int count = 0;
while (count < n) {
boolean found = false;
for (int i = 0; i < n; i++) {
if (!finish[i] && processes[i].canFinish(work)) {
finish[i] = true;
processes[i].finished = true;
for (int j = 0; j < m; j++) {
work[j] += processes[i].allocated[j];
}
found = true;
count++;
}
}
if (!found) {
return false;
}
}
return true;
}
// 试分配
boolean tryAllocate(Process process, int[] request) {
for (int i = 0; i < m; i++) {
if (request[i] > process.need[i]) {
System.out.println("Request exceeds need of process " + process.name);
return false;
}
if (request[i] > resource.available[i]) {
System.out.println("Request exceeds available resources");
return false;
}
}
process.allocate(request);
if (isSafe()) {
System.out.println("Allocation succeeded");
return true;
} else {
process.release(request);
System.out.println("Allocation failed, system would be unsafe");
return false;
}
}
// 运行函数
void run() {
Scanner scanner = new Scanner(System.in);
// 输入可用资源数目
System.out.print("Enter the available resources: ");
int[] available = new int[m];
for (int i = 0; i < m; i++) {
available[i] = scanner.nextInt();
}
resource.available = available;
// 安全性检查
if (isSafe()) {
System.out.println("The system is safe");
System.out.print("Safe sequence: ");
int[] work = available.clone();
boolean[] finish = new boolean[n];
for (int i = 0; i < n; i++) {
finish[i] = false;
}
int count = 0;
while (count < n) {
boolean found = false;
for (int i = 0; i < n; i++) {
if (!finish[i] && processes[i].canFinish(work)) {
finish[i] = true;
System.out.print(processes[i].name + " ");
for (int j = 0; j < m; j++) {
work[j] += processes[i].allocated[j];
}
found = true;
count++;
}
}
if (!found) {
break;
}
}
System.out.println();
} else {
System.out.println("The system is unsafe");
return;
}
// 试分配
while (true) {
System.out.print("Enter the name of process to request (or \"quit\" to exit): ");
String name = scanner.next();
if (name.equals("quit")) {
break;
}
System.out.print("Enter the request: ");
int[] request = new int[m];
for (int i = 0; i < m; i++) {
request[i] = scanner.nextInt();
}
Process process = null;
for (int i = 0; i < n; i++) {
if (processes[i].name.equals(name)) {
process = processes[i];
break;
}
}
if (process == null) {
System.out.println("Process not found");
continue;
}
tryAllocate(process, request);
}
}
public static void main(String[] args) {
BankersAlgorithm ba = new BankersAlgorithm();
ba.init();
ba.run();
}
}
```
在程序中,我们使用了`Process`类和`Resource`类来表示进程和资源,其中`Process`类包含了进程的名称、最大需求数目、已分配数目、仍需数目、是否完成和是否中止等属性,以及相关的方法,如打印进程信息、检查是否可以完成、分配资源和释放资源等。`Resource`类包含了资源的名称和当前状态下可利用数目。
在程序中,我们先输入所有初始化信息,包括进程数目、资源数目、进程的最大需求数目和已分配数目,以及资源的可用数目。输入完成后,程序会以矩阵的形式输出所有进程的相关信息。然后,程序会要求输入一组可用资源数目,并进行安全性检查,如果存在安全序列,程序会输出一个安全序列;否则,程序会提示初始化不正确并结束运行。
如果系统已经处于安全状态,程序可以接受某个进程的资源申请,并进行试分配。程序会检查用户提出的请求是否合法,如果请求合法,程序会进行试分配并调用安全性检查算法检查系统是否仍然处于安全状态。如果安全,程序会输出分配后的资源使用情况,并输出一个安全序列;否则,程序会拒绝申请并打印出相应的拒绝原因。
在程序中,我们使用了`Scanner`类来读取用户的输入,从而实现了与用户的交互。程序的主函数中,我们先调用`init`函数进行初始化,然后调用`run`函数进行运行。
阅读全文
相关推荐

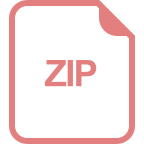
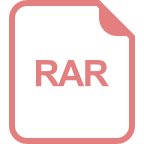
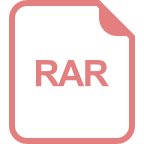
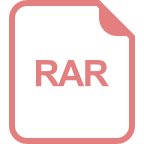
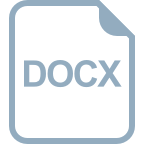
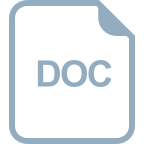
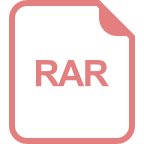
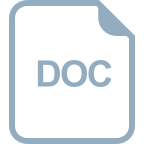
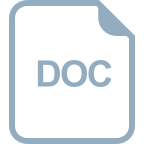
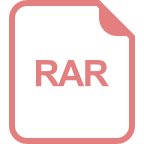
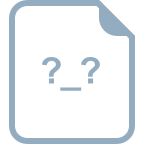
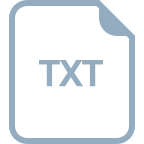
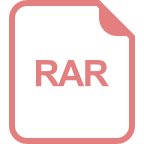