Flutter 中,数组对象转成map对象
时间: 2023-10-25 10:14:38 浏览: 142
在 Flutter 中,可以使用 `List` 类型的 `map` 方法来把数组对象转成 Map 对象。以下是示例代码:
```dart
void main() {
List<Map<String, dynamic>> list = [
{'id': 1, 'name': 'John'},
{'id': 2, 'name': 'Jane'},
{'id': 3, 'name': 'Bob'}
];
Map<int, String> map = Map.fromIterable(list,
key: (item) => item['id'], value: (item) => item['name']);
print(map);
}
```
在这个示例中,我们首先定义了一个 `List<Map<String, dynamic>>` 类型的数组对象 `list`,其中每个元素都是一个包含 `id` 和 `name` 两个属性的 Map 对象。
接着,我们使用 `Map.fromIterable` 方法来将数组对象 `list` 转成 Map 对象 `map`,其中 `key` 参数指定了如何获取每个元素的键值,即 `item['id']`,而 `value` 参数指定了如何获取每个元素的值,即 `item['name']`。
最后,我们通过 `print` 函数来打印转换后的 Map 对象 `map`,输出结果为 `{1: John, 2: Jane, 3: Bob}`。
相关问题
Flutter 中,数组对象转成map
在 Flutter 中,可以使用 `List` 类的 `asMap` 方法将一个数组对象转换为 `Map` 对象。该方法将原始数组的每个元素作为值,并将其索引作为键。
以下是一个示例代码:
```dart
List<String> fruits = ['apple', 'banana', 'orange'];
Map<int, String> fruitMap = fruits.asMap();
print(fruitMap); // {0: 'apple', 1: 'banana', 2: 'orange'}
```
在上面的示例中,`List<String>` 对象 `fruits` 被转换为一个 `Map<int, String>` 对象 `fruitMap`,其中键是整数类型的索引,值是字符串类型的元素值。
注意,`asMap` 方法返回的是一个不可变的 `Map` 对象,如果需要对其进行修改,可以使用 `Map` 类的 `addAll` 方法将其内容复制到一个新的可变 `Map` 对象中,并进行修改。
flutter怎么把数组对象转成map对象再转成数组对象
在Dart语言中,可以使用map()方法将List对象转换为Map对象,然后使用toList()方法将Map对象转换为List对象。
以下是一个示例代码:
```dart
class Person {
String name;
int age;
Person(this.name, this.age);
}
void main() {
List<Person> persons = [
Person('Alice', 25),
Person('Bob', 30),
Person('Charlie', 35)
];
// 将List<Person>转换为Map<String, dynamic>
Map<String, dynamic> personMap = Map.fromIterable(persons, key: (p) => p.name, value: (p) => {'age': p.age});
// 将Map<String, dynamic>转换为List<Map<String, dynamic>>
List<Map<String, dynamic>> personList = personMap.values.toList();
print(personList);
}
```
在上面的代码中,我们定义了一个Person类,然后创建了一个包含三个Person对象的List。接着,我们使用fromIterable()方法将List<Person>转换为Map<String, dynamic>,其中key是Person对象的name属性,value是一个包含age属性的Map对象。然后,我们再使用values.toList()方法将Map<String, dynamic>转换为List<Map<String, dynamic>>,即包含三个Map对象的List。
阅读全文
相关推荐
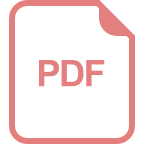
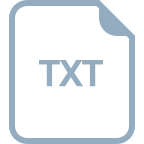
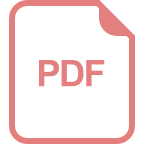








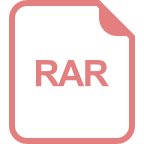
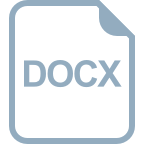
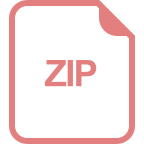
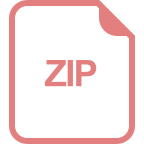
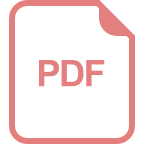
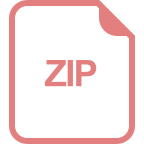