qt实现spy++功能
时间: 2023-10-23 07:15:06 浏览: 220
Spy 是一款 Windows 平台上的工具,用于查看和调试其他应用程序的窗口和消息。在 Qt 中,可以使用 QWindow 类和 QEvent 类来实现类似的功能。下面是一个简单的示例代码,可以实现查看其他应用程序窗口的功能:
```cpp
#include <QtWidgets>
class WindowSpy : public QWidget
{
public:
WindowSpy(QWidget *parent = nullptr) : QWidget(parent)
{
setWindowTitle("Window Spy");
setFixedSize(400, 300);
QVBoxLayout *layout = new QVBoxLayout(this);
QLabel *label = new QLabel("Click on a window to spy on it.", this);
label->setAlignment(Qt::AlignCenter);
layout->addWidget(label);
m_textEdit = new QTextEdit(this);
layout->addWidget(m_textEdit);
// 安装事件过滤器,以便捕获鼠标点击事件
qApp->installEventFilter(this);
}
protected:
bool eventFilter(QObject *obj, QEvent *event) override
{
if (event->type() == QEvent::MouseButtonPress)
{
QMouseEvent *mouseEvent = static_cast<QMouseEvent *>(event);
// 获取鼠标点击的窗口
QWidget *widget = QApplication::widgetAt(mouseEvent->globalPos());
if (widget)
{
// 获取窗口信息并显示
QString info = QString("Window: %1\nClass: %2\n").arg(widget->windowTitle(), widget->metaObject()->className());
m_textEdit->setText(info);
}
}
return QWidget::eventFilter(obj, event);
}
private:
QTextEdit *m_textEdit;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
WindowSpy windowSpy;
windowSpy.show();
return app.exec();
}
```
这个示例代码中,我们创建了一个窗口,当用户点击其他应用程序的窗口时,会显示该窗口的信息,包括窗口名称和类名。使用 `QApplication::widgetAt()` 函数可以获取鼠标点击的窗口,然后使用 `metaObject()` 函数获取窗口的类名。如果需要进一步查看窗口的消息,可以使用 `QWindow` 类和 `QEvent` 类来实现。
阅读全文
相关推荐
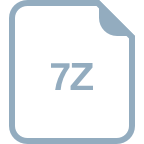
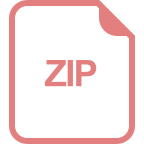
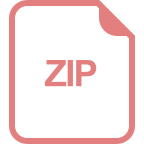















