c#datagridview获取选中行
时间: 2023-06-05 10:47:24 浏览: 341
在 C# 中使用 DataGridView 控件时,可以使用 SelectedRows 属性来获取选中的行。示例代码如下:
```
foreach (DataGridViewRow row in dataGridView1.SelectedRows)
{
Console.WriteLine(row.Cells[0].Value.ToString());
}
```
这段代码将遍历所有选中的行,并输出每行的第一列的值。
另外还可以使用下面的方法来获取选中的单行
```
DataGridViewRow selectedRow = dataGridView1.Rows[dataGridView1.SelectedCells[0].RowIndex];
```
这段代码将获取单行的选中的单元格的行索引对应的行。
相关问题
C#dataGridView改变选中行颜色
在C#中,你可以通过 dataGridView 的 `DefaultCellStyle` 或 `SelectionColor` 属性来改变 DataGridView 中选中行的颜色。以下是一个简单的示例:
```csharp
// 获取当前的默认选中颜色
DataGridViewCellStyle defaultCellStyle = dataGridViewCellStyle.DefaultCellStyle;
// 如果你想更改整个表格的选中颜色,可以这样做:
dataGridView.DefaultCellStyle.SelectionBackColor = Color.Yellow; // 设置成黄色作为示例
// 如果只想针对特定列,比如第一列(列索引从0开始计数),则:
dataGridView.Columns[0].DefaultCellStyle.SelectionBackColor = Color.Blue;
// 当然,如果你想在某一行被选中时动态更改颜色,可以在 `CellValueChanged` 或 `RowHeaderMouseClick` 等事件中设置:
private void dataGridView_CellValueChanged(object sender, DataGridViewCellEventArgs e)
{
if (dataGridView.CurrentRow.Selected) // 判断是否被选中
{
dataGridView.CurrentRow.DefaultCellStyle.BackColor = Color.Gray; // 设置背景色
}
}
C# datagridview 设定选中行的边框
在C#的DataGridView控件中,如果你想设定选中行的边框样式,可以使用`DefaultCellStyle`属性以及`SelectionBorder`属性来进行设置。首先,你需要获取或创建` DataGridViewCellStyle`对象,然后设置它的` BorderStyle`属性来定义边框样式,最后将这个样式应用到选中行上。
以下是简单的步骤:
```csharp
// 获取当前DefaultCellStyle
DataGridViewCellStyle dataGridViewCellStyle = dataGridView.DefaultCellStyle;
// 设置边框样式,例如,SolidLine表示实线边框
dataGrid.DefaultCellStyle.SelectionBorder = DataGridViewSelectionBorder.SolidLine;
// 如果你想只对某特定列应用此样式,可以这样做:
dataGridView.Columns["YourColumnHeader"].DefaultCellStyle.SelectionBorder = DataGridViewSelectionBorder.SolidLine;
// 或者你可以直接设置选中的单元格的样式:
dataGridView.Rows[selectedIndex].DefaultCellStyle.SelectionBackColor = Color.Gray; // 选中背景色
dataGridView.Rows[selectedIndex].DefaultCellStyle.SelectionForeColor = Color.Black; // 选中文本颜色
// 应用新的样式
dataGridView.SelectedRowsDefaultCellStyle = dataGridViewCellStyle;
```
请注意替换"YourColumnHeader"为你的实际列名,并将`selectedIndex`替换为你想要高亮显示的行的索引。
阅读全文
相关推荐
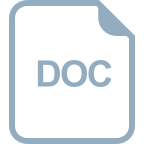



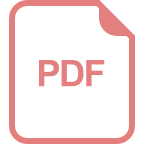
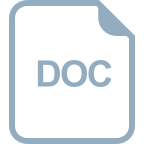
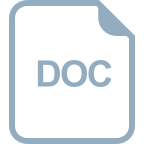
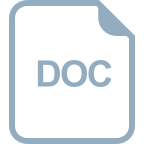





