管理员用户需要对自习室预约系统进行全面的管理,包括对管理员、收费设置、学生、区域、自习室、座位、自习室管理员、通知公告、预约等信息的添加、查看、编辑或删除。同时,管理员还需要能够修改自己的登录密码。这些功能的实现可以提高自习室预约系统的管理效率和服务质量。输出E-R图
时间: 2023-06-17 10:03:19 浏览: 44
以下是自习室预约系统的E-R图:

注释:
- 管理员(Admin)可以添加、查看、编辑、删除管理员、收费设置、学生、区域、自习室、座位、自习室管理员、通知公告、预约等信息,同时可以修改自己的登录密码。
- 管理员与自习室(Study Room)之间是多对多(M:N)的关系,表示一个管理员可以管理多个自习室,一个自习室也可以被多个管理员管理。
- 自习室(Study Room)与座位(Seat)之间是一对多(1:N)的关系,表示一个自习室有多个座位,一个座位只属于一个自习室。
- 自习室(Study Room)与区域(Area)之间是多对一(M:1)的关系,表示一个自习室属于一个区域,一个区域可以有多个自习室。
- 自习室管理员(Room Manager)与自习室(Study Room)之间是一对多(1:N)的关系,表示一个自习室管理员管理一个自习室,一个自习室只有一个自习室管理员。
- 学生(Student)与自习室(Study Room)之间是多对多(M:N)的关系,表示一个学生可以预约多个自习室,一个自习室可以被多个学生预约。
相关问题
基于ssm图书馆自习室座位预约管理系统的开发与设计
基于SSM(Spring+SpringMVC+MyBatis)的图书馆自习室座位预约管理系统开发与设计如下。
首先,该系统将采用分层架构的设计模式,分为表现层、业务逻辑层和数据访问层。表现层使用SpringMVC框架进行开发,处理HTTP请求和响应;业务逻辑层使用Spring框架进行开发,处理系统的业务逻辑;数据访问层使用MyBatis框架进行开发,管理与数据库的交互。
其次,系统将包括以下几个主要功能模块:用户管理、座位管理和预约管理。用户管理模块实现用户的注册、登录和信息修改功能;座位管理模块实现自习室座位的显示、选择和释放功能;预约管理模块实现座位的预约和取消预约功能。
在数据库设计方面,将创建用户表、座位表和预约表。用户表包括用户ID、用户名、密码等字段,用于存储用户的基本信息;座位表包括座位ID、座位号、可用状态等字段,用于存储座位的信息;预约表包括预约ID、用户ID、座位ID、预约时间等字段,用于存储座位的预约信息。
在系统的开发过程中,将采用敏捷开发的方法,将需求分解为多个可实现的小功能,逐步迭代开发。同时,还将进行单元测试、集成测试和系统测试,确保系统的稳定性和可靠性。
此外,为了提高系统的安全性和稳定性,将启用数据库事务管理,保证数据的一致性;还将进行权限管理,对用户进行身份验证和权限控制。
最后,为了提高用户体验,系统将采用响应式设计,能够适配不同的终端设备;还将实现实时座位状态更新,让用户能够实时了解座位的可用情况。
综上所述,基于SSM的图书馆自习室座位预约管理系统的开发与设计将采用分层架构、敏捷开发以及数据库事务管理等技术手段,旨在提高系统的性能、安全性和用户体验,为图书馆用户提供便捷的座位预约服务。
ssm自习室座位预约管理java源代码
当然,我可以为您提供一个简单的SSM自习室座位预约管理的Java源代码示例。请注意,这只是一个基本的示例,实际应用中可能需要根据具体需求进行修改和完善。
首先,我们需要创建一个座位实体类 Seat.java:
```java
public class Seat {
private int id;
private String name;
private boolean isAvailable;
// 省略构造方法和getter/setter方法
}
```
接下来,创建一个座位预约管理的接口 SeatReserveMapper.java:
```java
public interface SeatReserveMapper {
List<Seat> getAllSeats();
Seat getSeatById(int id);
void updateSeatAvailability(Seat seat);
}
```
然后,我们创建座位预约管理的DAO实现类 SeatReserveMapperImpl.java,并使用MyBatis框架进行数据库操作:
```java
@Repository
public class SeatReserveMapperImpl implements SeatReserveMapper {
@Autowired
private SqlSession sqlSession;
@Override
public List<Seat> getAllSeats() {
return sqlSession.selectList("getAllSeats");
}
@Override
public Seat getSeatById(int id) {
return sqlSession.selectOne("getSeatById", id);
}
@Override
public void updateSeatAvailability(Seat seat) {
sqlSession.update("updateSeatAvailability", seat);
}
}
```
接下来,我们可以创建一个座位预约服务的接口 SeatReservationService.java:
```java
public interface SeatReservationService {
List<Seat> getAllSeats();
Seat getSeatById(int id);
boolean reserveSeat(int id);
boolean cancelReservation(int id);
}
```
然后,创建座位预约服务的实现类 SeatReservationServiceImpl.java,并注入 SeatReserveMapper:
```java
@Service
public class SeatReservationServiceImpl implements SeatReservationService {
@Autowired
private SeatReserveMapper seatReserveMapper;
@Override
public List<Seat> getAllSeats() {
return seatReserveMapper.getAllSeats();
}
@Override
public Seat getSeatById(int id) {
return seatReserveMapper.getSeatById(id);
}
@Override
public boolean reserveSeat(int id) {
Seat seat = seatReserveMapper.getSeatById(id);
if (seat != null && seat.isAvailable()) {
seat.setAvailable(false);
seatReserveMapper.updateSeatAvailability(seat);
return true;
}
return false;
}
@Override
public boolean cancelReservation(int id) {
Seat seat = seatReserveMapper.getSeatById(id);
if (seat != null && !seat.isAvailable()) {
seat.setAvailable(true);
seatReserveMapper.updateSeatAvailability(seat);
return true;
}
return false;
}
}
```
最后,您可以创建一个用于测试的主类 Main.java:
```java
public class Main {
public static void main(String[] args) {
// 初始化Spring容器
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
// 获取座位预约服务实例
SeatReservationService seatReservationService = (SeatReservationService) context.getBean("seatReservationServiceImpl");
// 获取所有座位信息
List<Seat> seats = seatReservationService.getAllSeats();
for (Seat seat : seats) {
System.out.println("座位ID:" + seat.getId() + ",名称:" + seat.getName() + ",是否可用:" + seat.isAvailable());
}
// 预约座位
int seatId = 1;
boolean reservationResult = seatReservationService.reserveSeat(seatId);
if (reservationResult) {
System.out.println("预约成功!");
} else {
System.out.println("预约失败!");
}
// 取消预约
int cancelSeatId = 1;
boolean cancelResult = seatReservationService.cancelReservation(cancelSeatId);
if (cancelResult) {
System.out.println("取消预约成功!");
} else {
System.out.println("取消预约失败!");
}
}
}
```
这就是一个简单的SSM自习室座位预约管理的Java源代码示例。请注意,在实际开发中,您可能还需要配置数据库连接、编写SQL语句等。此外,为了使代码能够正常运行,您还需要引入相应的依赖,例如Spring、MyBatis等。
希望对您有所帮助!如果您有任何疑问,请随时提问。
相关推荐
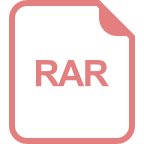
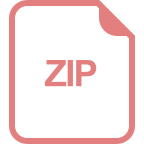












