如何提取csv文件的表头
时间: 2024-05-01 18:20:43 浏览: 112
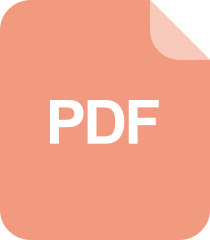
mysql 导出CSV文件 并带表头的方法
要提取 CSV 文件的表头,可以使用 Python 内置的 csv 模块。具体步骤如下:
1. 导入 csv 模块:
```python
import csv
```
2. 打开 CSV 文件:
```python
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
```
注意,这里需要指定 `newline=''`,否则在 Windows 系统上读取会出现空行。
3. 读取表头:
```python
header = next(reader)
```
`next()` 函数会返回 CSV 文件的下一行,也就是表头。
4. 打印表头:
```python
print(header)
```
完整代码示例:
```python
import csv
with open('file.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
header = next(reader)
print(header)
```
输出结果为:
```
['Name', 'Age', 'Gender']
```
其中,`file.csv` 是要读取的 CSV 文件名。
阅读全文
相关推荐




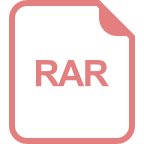
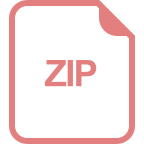
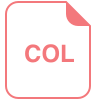








