从键盘输入某班学生某门课的成绩(每班人数最多不超过40人),当输入为负值时,表示输入结束,试编程计算最高分(编写自定义函数实现)。 **输入格式要求:"%d" 提示信息:"Total students are %d\n" "The highest score is %d\n" "Input score:" **输出格式要求:"%d"
时间: 2023-07-05 22:24:01 浏览: 168
好的,这是一个简单的C语言编程问题。下面是代码实现:
```c
#include <stdio.h>
int highest_score(int scores[], int num_students) {
int max_score = scores[0];
for (int i = 1; i < num_students; i++) {
if (scores[i] > max_score) {
max_score = scores[i];
}
}
return max_score;
}
int main() {
int scores[40];
int num_students = 0;
int score = 0;
printf("Total students are %d\n", 40);
printf("Input score: ");
while (scanf("%d", &score) == 1 && score >= 0 && num_students < 40) {
scores[num_students] = score;
num_students++;
printf("Input score: ");
}
int max_score = highest_score(scores, num_students);
printf("The highest score is %d\n", max_score);
return 0;
}
```
这个程序定义了一个 `highest_score` 函数,用于计算最高分。在 `main` 函数中,先定义了一个长度为40的数组 `scores`,以及一个变量 `num_students` 表示输入的学生人数。然后通过 `while` 循环不断从键盘输入学生成绩,直到输入负值或者已经输入了40个学生的成绩为止。
每次输入一个成绩,就将其保存到数组 `scores` 中,并将 `num_students` 加1。然后再提示输入下一个成绩,直到输入结束。
最后调用 `highest_score` 函数,计算出最高分并输出即可。
相关推荐
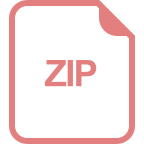
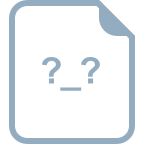
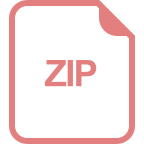














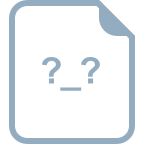
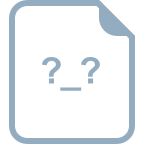