请帮我提供一个JAVAV具体是如何实现Excel导入导出的具体案例
时间: 2023-05-24 20:02:07 浏览: 100
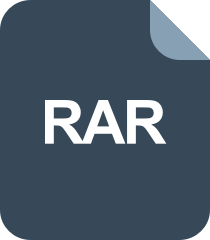
java excel导入导出的示例
当然,我可以为您提供一个Java实现Excel导入导出的具体案例。
首先,我们需要导入 Apache POI 库来操作Excel文件,然后在代码中导入相应的包:
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.BorderStyle;
然后是具体的实现代码:
// 导入Excel文件
public Workbook importExcel(InputStream is) throws Exception {
Workbook workbook = WorkbookFactory.create(is);
return workbook;
}
// 导出Excel文件
public void exportExcel(List data, OutputStream os) throws Exception {
Workbook workbook = new XSSFWorkbook();
Sheet sheet = workbook.createSheet("Sheet1");
// 设置表头
Row header = sheet.createRow(0);
CellStyle headerStyle = workbook.createCellStyle();
headerStyle.setAlignment(HorizontalAlignment.CENTER);
Font headerFont = workbook.createFont();
headerFont.setBold(true);
headerFont.setColor(IndexedColors.WHITE.getIndex());
headerStyle.setFillForegroundColor(IndexedColors.SEA_GREEN.getIndex());
headerStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND);
headerStyle.setFont(headerFont);
String[] headerTitles = {"ID", "名称", "分数"};
for (int i = 0; i < headerTitles.length; i++) {
Cell cell = header.createCell(i);
cell.setCellValue(headerTitles[i]);
cell.setCellStyle(headerStyle);
}
// 设置表格内容
CellStyle contentStyle = workbook.createCellStyle();
contentStyle.setAlignment(HorizontalAlignment.CENTER);
Font contentFont = workbook.createFont();
contentFont.setColor(IndexedColors.AUTOMATIC.getIndex());
contentStyle.setFont(contentFont);
int rownum = 1;
for (Object obj : data) {
Row row = sheet.createRow(rownum++);
if (obj instanceof Student) {
Student s = (Student) obj;
Cell cell0 = row.createCell(0);
cell0.setCellValue(s.getId());
cell0.setCellStyle(contentStyle);
Cell cell1 = row.createCell(1);
cell1.setCellValue(s.getName());
cell1.setCellStyle(contentStyle);
Cell cell2 = row.createCell(2);
cell2.setCellValue(s.getScore());
cell2.setCellStyle(contentStyle);
}
}
// 调整列宽
sheet.autoSizeColumn(0);
sheet.autoSizeColumn(1);
sheet.autoSizeColumn(2);
// 导出Excel文件
workbook.write(os);
workbook.close();
}
这是一个非常简单的案例,有关Excel导入导出更多的内容可以查询Apache POI官方文档。
阅读全文
相关推荐
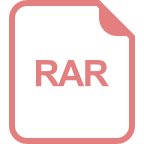

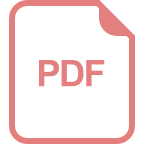
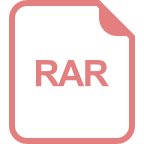
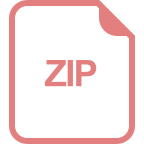
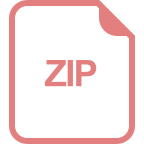
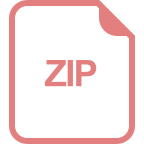
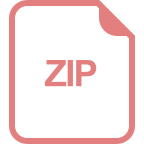







