将容器中的点拟合成四条或三条直线C++
时间: 2024-03-06 22:49:09 浏览: 27
要将容器中的点拟合成四条或三条直线,可以使用霍夫变换算法。以下是使用OpenCV库实现的C++代码示例:
```c++
#include <opencv2/opencv.hpp>
#include <vector>
using namespace std;
using namespace cv;
void fitLines(vector<Point>& points, int numLines, vector<Vec4f>& lines) {
Mat pointsMat(points);
float rho = 1; // distance resolution in pixels
float theta = CV_PI / 180; // angle resolution in radians
float threshold = 50; // minimum number of intersections to detect a line
float minLineLength = 50; // minimum length of a line
float maxLineGap = 10; // maximum allowed gap between points on the same line
HoughLinesP(pointsMat, lines, rho, theta, threshold, minLineLength, maxLineGap);
// sort lines by their length
sort(lines.begin(), lines.end(), [](const Vec4f& line1, const Vec4f& line2) {
return norm(Point(line1[0], line1[1]) - Point(line1[2], line1[3])) >
norm(Point(line2[0], line2[1]) - Point(line2[2], line2[3]));
});
// keep only the first numLines lines
lines.resize(numLines);
}
int main() {
vector<Point> points = {Point(0, 0), Point(10, 10), Point(20, 0), Point(30, 10), Point(40, 0)};
vector<Vec4f> lines;
fitLines(points, 2, lines);
for (const auto& line : lines) {
cout << "line: (" << line[0] << "," << line[1] << ") - ("
<< line[2] << "," << line[3] << ")" << endl;
}
return 0;
}
```
这里的`points`是输入的点集,`numLines`是要拟合的直线条数,`lines`是输出的直线参数。在函数`fitLines`中,我们使用了OpenCV的霍夫变换函数`HoughLinesP`来得到直线参数。然后按照直线长度从大到小排序,取前`numLines`条直线即可。
相关推荐
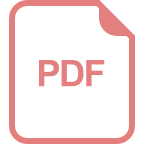
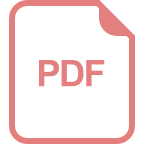
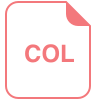
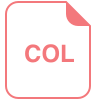
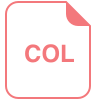
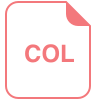
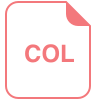









