请用C#设计一个测试引擎,能够支持串行测试、并行测试、条件测试。测试项包含校准项和验证项,该测试引擎需要支持“先测后校”的逻辑:跳过所有校准项,先测试验证项;当验证项失败时,可以触发对应的校准项进行测试。
时间: 2024-01-16 09:03:48 浏览: 22
以下是使用C#设计的测试引擎,支持串行测试、并行测试、条件测试,并且支持“先测后校”的逻辑:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace TestEngine
{
public class TestEngine
{
private List<TestItem> _testItems;
private List<TestItem> _calibrationItems;
private TestItem _currentTestItem;
private TestItem _currentCalibrationItem;
public TestEngine(List<TestItem> testItems, List<TestItem> calibrationItems)
{
_testItems = testItems;
_calibrationItems = calibrationItems;
}
public async Task RunAsync(ScheduleType scheduleType)
{
if (scheduleType == ScheduleType.Serial)
{
await RunSerialAsync();
}
else if (scheduleType == ScheduleType.Parallel)
{
await RunParallelAsync();
}
else if (scheduleType == ScheduleType.Conditional)
{
await RunConditionalAsync();
}
}
private async Task RunSerialAsync()
{
foreach (var testItem in _testItems)
{
_currentTestItem = testItem;
await RunTestItemAsync(testItem);
}
foreach (var calibrationItem in _calibrationItems)
{
_currentCalibrationItem = calibrationItem;
await RunTestItemAsync(calibrationItem);
}
}
private async Task RunParallelAsync()
{
var tasks = new List<Task>();
foreach (var testItem in _testItems)
{
_currentTestItem = testItem;
tasks.Add(RunTestItemAsync(testItem));
}
await Task.WhenAll(tasks);
tasks.Clear();
foreach (var calibrationItem in _calibrationItems)
{
_currentCalibrationItem = calibrationItem;
tasks.Add(RunTestItemAsync(calibrationItem));
}
await Task.WhenAll(tasks);
}
private async Task RunConditionalAsync()
{
var tasks = new List<Task>();
foreach (var testItem in _testItems)
{
_currentTestItem = testItem;
tasks.Add(RunTestItemAsync(testItem));
}
await Task.WhenAll(tasks);
if (!_testItems.All(ti => ti.Passed))
{
var failedTestItems = _testItems.Where(ti => !ti.Passed);
var calibrationItem = _calibrationItems.FirstOrDefault(ci => ci.CanCalibrate(failedTestItems));
if (calibrationItem != null)
{
_currentCalibrationItem = calibrationItem;
await RunTestItemAsync(calibrationItem);
}
}
}
private async Task RunTestItemAsync(TestItem testItem)
{
await Task.Run(() =>
{
testItem.Run();
if (!testItem.Passed && testItem.CanCalibrate(_currentTestItem))
{
var calibrationItem = _calibrationItems.FirstOrDefault(ci => ci.CanCalibrate(_currentTestItem));
if (calibrationItem != null)
{
_currentCalibrationItem = calibrationItem;
calibrationItem.Run();
}
}
});
}
}
public enum ScheduleType
{
Serial,
Parallel,
Conditional
}
public abstract class TestItem
{
public string Name { get; set; }
public bool Passed { get; set; }
public abstract void Run();
public virtual bool CanCalibrate(TestItem failedTestItem)
{
return false;
}
}
public class CalibrationItem : TestItem
{
private Func<TestItem, bool> _calibrationCondition;
public CalibrationItem(string name, Func<TestItem, bool> calibrationCondition)
{
Name = name;
_calibrationCondition = calibrationCondition;
}
public override void Run()
{
Console.WriteLine($"Running calibration item: {Name}");
Passed = true;
}
public override bool CanCalibrate(TestItem failedTestItem)
{
return _calibrationCondition(failedTestItem);
}
}
public class ValidationItem : TestItem
{
private Func<TestItem, bool> _calibrationCondition;
public ValidationItem(string name, Func<TestItem, bool> calibrationCondition)
{
Name = name;
_calibrationCondition = calibrationCondition;
}
public override void Run()
{
Console.WriteLine($"Running validation item: {Name}");
Passed = true;
}
public override bool CanCalibrate(TestItem failedTestItem)
{
return _calibrationCondition(failedTestItem);
}
}
}
```
在这个测试引擎中,`TestEngine` 类包含了 `RunAsync` 方法,根据传入的 `ScheduleType` 参数,分别调用 `RunSerialAsync`、`RunParallelAsync`、`RunConditionalAsync` 方法执行测试。
`RunSerialAsync` 方法按照顺序执行测试项和校准项;`RunParallelAsync` 方法并行执行测试项和校准项;`RunConditionalAsync` 方法先执行所有测试项,如果有测试项失败,则根据校准项的条件选择一个校准项进行测试。
`TestItem` 是测试项的基类,包含了测试项的名称和是否测试通过等属性,以及 `Run` 和 `CanCalibrate` 方法。`Run` 方法用于执行测试,`CanCalibrate` 方法用于判断当前测试项是否需要进行校准。
`CalibrationItem` 和 `ValidationItem` 分别是校准项和验证项的子类,它们继承了 `TestItem` 类,重写了 `Run` 和 `CanCalibrate` 方法。在这个例子中,校准项和验证项都包含一个 `Func<TestItem, bool>` 类型的变量 `_calibrationCondition`,用于指定在哪些情况下需要进行校准。
使用这个测试引擎,可以按照以下方式进行测试:
```csharp
var testItems = new List<TestItem>
{
new ValidationItem("Test 1", ti => true),
new ValidationItem("Test 2", ti => true),
new ValidationItem("Test 3", ti => true),
new ValidationItem("Test 4", ti => true)
};
var calibrationItems = new List<TestItem>
{
new CalibrationItem("Calibration 1", ti => ti.Name == "Test 1" || ti.Name == "Test 2"),
new CalibrationItem("Calibration 2", ti => ti.Name == "Test 3" || ti.Name == "Test 4")
};
var testEngine = new TestEngine(testItems, calibrationItems);
await testEngine.RunAsync(ScheduleType.Conditional);
```
在这个例子中,创建了四个测试项和两个校准项,使用 `TestEngine` 类执行了条件测试。其中,`Test 1` 和 `Test 2` 是需要校准的测试项,`Calibration 1` 是校准 `Test 1` 和 `Test 2` 的校准项,`Test 3` 和 `Test 4` 是需要校准的测试项,`Calibration 2` 是校准 `Test 3` 和 `Test 4` 的校准项。
相关推荐
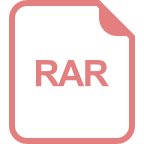
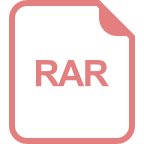
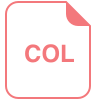

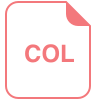
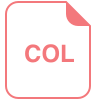
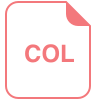









