将文件读入结构体数组中并运用
时间: 2024-03-01 19:56:02 浏览: 89
好的,我可以帮你解答这个问题。首先,你需要定义一个结构体,该结构体应该包含你想要在文件中存储的数据类型。例如,如果你想要从文件中读取学生的姓名、年龄和分数,那么你可以定义如下的结构体:
```c
typedef struct {
char name[20];
int age;
float score;
} Student;
```
接下来,你需要打开文件并读取数据。你可以使用`fscanf`函数从文件中读取数据,并将其存储在结构体数组中。假设你的文件名为`data.txt`,你可以使用如下的代码将文件中的数据读入到结构体数组中:
```c
#include <stdio.h>
int main() {
FILE *fp;
Student students[100];
int count = 0;
fp = fopen("data.txt", "r");
if (fp == NULL) {
printf("Failed to open file!\n");
return 1;
}
while (fscanf(fp, "%s %d %f", students[count].name, &students[count].age, &students[count].score) == 3) {
count++;
}
fclose(fp);
// 现在你可以使用数据了
for (int i = 0; i < count; i++) {
printf("Name: %s, Age: %d, Score: %.2f\n", students[i].name, students[i].age, students[i].score);
}
return 0;
}
```
在上面的代码中,我们首先打开了`data.txt`文件并检查是否成功。然后,我们使用`fscanf`函数从文件中读取数据,并将其存储在结构体数组`students`中。每次成功读取一行数据时,我们将计数器`count`增加1。最后,我们使用一个循环遍历结构体数组中的数据,并将其打印出来。
希望这可以回答你的问题。如果你还有其他问题,请随时问我。
阅读全文
相关推荐
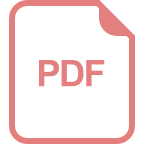
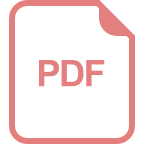
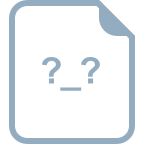















