用c++做一个学生成绩系统,要能实现学生成绩表的建立、插入、删除、查找、总分的排序的基本操作的实现
时间: 2023-12-21 17:05:11 浏览: 83
好的,这是一个非常典型的基于数据结构的应用,以下是一个简单的 C++ 实现:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
struct Student {
int id;
std::string name;
int score1;
int score2;
int score3;
int total;
double average;
Student(int id_, std::string name_, int score1_, int score2_, int score3_)
: id(id_), name(name_), score1(score1_), score2(score2_), score3(score3_), total(score1_ + score2_ + score3_), average(total / 3.0) {}
};
class StudentSystem {
public:
void insert(int id, std::string name, int score1, int score2, int score3) {
students.push_back(Student(id, name, score1, score2, score3));
}
void remove(int id) {
for (auto it = students.begin(); it != students.end(); it++) {
if (it->id == id) {
students.erase(it);
return;
}
}
}
Student* find(int id) {
for (auto& student : students) {
if (student.id == id) {
return &student;
}
}
return nullptr;
}
void sort() {
std::sort(students.begin(), students.end(), [](const Student& a, const Student& b) {
return a.total > b.total;
});
}
void print() {
std::cout << "ID\tName\tScore1\tScore2\tScore3\tTotal\tAverage" << std::endl;
for (const auto& student : students) {
std::cout << student.id << "\t" << student.name << "\t" << student.score1 << "\t" << student.score2 << "\t" << student.score3 << "\t" << student.total << "\t" << student.average << std::endl;
}
}
private:
std::vector<Student> students;
};
int main() {
StudentSystem system;
// test insert, remove, find
system.insert(1, "Alice", 90, 80, 70);
system.insert(2, "Bob", 80, 70, 60);
system.insert(3, "Charlie", 70, 60, 50);
system.print();
system.remove(2);
system.print();
auto student = system.find(3);
if (student) {
std::cout << "Found student: " << student->name << std::endl;
} else {
std::cout << "Student not found" << std::endl;
}
// test sort
system.insert(4, "David", 60, 50, 40);
system.insert(5, "Eva", 50, 40, 30);
system.sort();
system.print();
return 0;
}
```
在这个实现中,`Student` 表示一个学生对象,包含学号、姓名和三科成绩,以及计算得到的总分和平均分。`StudentSystem` 表示学生成绩系统,支持插入、删除、查找、排序和打印学生成绩表的操作。具体实现细节见代码注释。
阅读全文
相关推荐
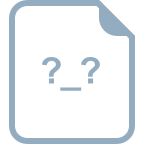



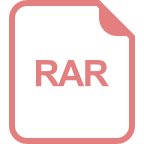
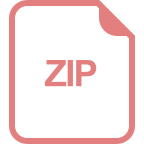
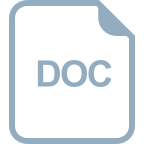
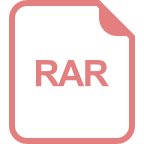
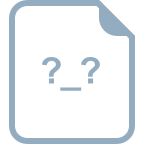
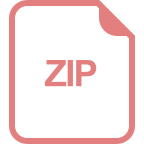
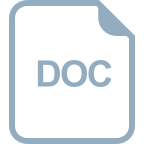
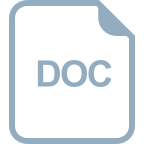
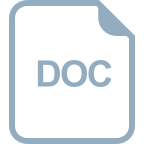
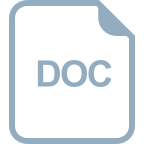
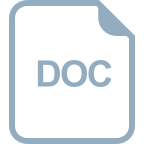
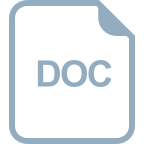
