java提供一个能体现可见性的例子
时间: 2023-10-21 19:03:54 浏览: 84
一个简单的例子是多线程中对共享变量的访问。假设有一个共享变量x,多个线程可能会同时对它进行读写操作。如果没有正确使用同步机制,就可能出现可见性问题。
例如,一个线程可能将x的值修改了,但是另一个线程并没有看到这个修改。这是因为线程之间的缓存不一定是同步的,一个线程对变量的修改可能还没有被刷新到主存中,另一个线程就已经从自己的缓存中读取了这个变量,导致出现不一致的结果。
下面是一个简单的示例代码,展示了可见性问题的出现:
```
class VisibilityDemo {
private static boolean flag = true;
public static void main(String[] args) throws InterruptedException {
new Thread(() -> {
while (flag) {
System.out.println("Thread 1 is running");
}
System.out.println("Thread 1 is done");
}).start();
Thread.sleep(1000);
flag = false;
System.out.println("Main thread sets flag to false");
}
}
```
该代码启动了两个线程,一个线程不断打印信息,另一个线程修改共享变量flag的值为false。由于没有使用同步机制来保证可见性,因此第一个线程可能无法看到flag的修改,导致它无法退出循环。
可以通过使用volatile关键字来解决可见性问题,它可以确保变量的修改被立即刷新到主存中,从而保证其他线程可以看到最新的值。修改后的代码如下:
```
class VisibilityDemo {
private static volatile boolean flag = true;
public static void main(String[] args) throws InterruptedException {
new Thread(() -> {
while (flag) {
System.out.println("Thread 1 is running");
}
System.out.println("Thread 1 is done");
}).start();
Thread.sleep(1000);
flag = false;
System.out.println("Main thread sets flag to false");
}
}
```
在这个版本中,flag变量的声明加上了volatile关键字,保证了可见性。运行该代码,可以看到第一个线程可以正确退出循环。
阅读全文
相关推荐
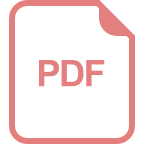
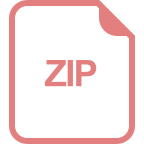
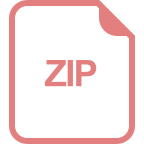
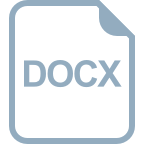
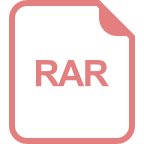
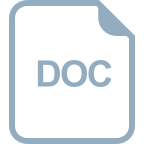
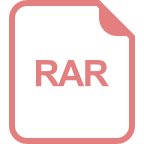
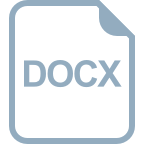
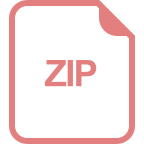
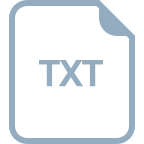
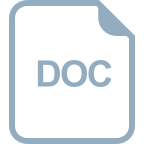
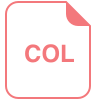
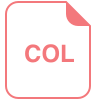
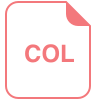
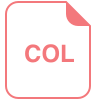
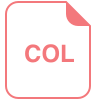