def remove_colors_except(image, target_colors, threshold): result = np.zeros_like(image) for target_color in target_colors: # 将目标色格式 "FFFFFF" 转换成 RGB 值 blue = int(target_color[0:2], 16) green = int(target_color[2:4], 16) red = int(target_color[4:6], 16) # 计算目标颜色的范围 lower_range = np.array([blue - threshold, green - threshold, red - threshold]) upper_range = np.array([blue + threshold, green + threshold, red + threshold]) # 创建掩码,将目标颜色范围以外的像素设为黑色,目标颜色范围内的像素设为白色 mask = cv2.inRange(image, lower_range, upper_range) # 将目标颜色范围内的像素设为白色 result[mask > 0] = (255, 255, 255) # 显示结果图像 cv2.imwrite('222.bmp', result) return result 修改可以传入多个颜色
时间: 2024-02-14 16:16:03 浏览: 65
可以将 `target_colors` 参数修改为一个列表,存储多个颜色值。然后在 `for` 循环中遍历这个列表,对每个颜色值进行相同的操作即可。具体代码如下:
```
def remove_colors_except(image, target_colors, threshold):
result = np.zeros_like(image)
for target_color in target_colors:
# 将目标色格式 "FFFFFF" 转换成 RGB 值
blue = int(target_color[0:2], 16)
green = int(target_color[2:4], 16)
red = int(target_color[4:6], 16)
# 计算目标颜色的范围
lower_range = np.array([blue - threshold, green - threshold, red - threshold])
upper_range = np.array([blue + threshold, green + threshold, red + threshold])
# 创建掩码,将目标颜色范围以外的像素设为黑色,目标颜色范围内的像素设为白色
mask = cv2.inRange(image, lower_range, upper_range)
# 将目标颜色范围内的像素设为白色
result[mask > 0] = (255, 255, 255)
# 显示结果图像
cv2.imwrite('222.bmp', result)
return result
```
调用函数时,可以传入一个颜色值列表,例如 `target_colors = ['#FF0000', '#00FF00', '#0000FF']`。函数会对每个颜色值分别进行操作,最终返回一个合并了所有颜色的结果图像。
阅读全文
相关推荐
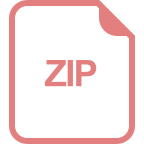
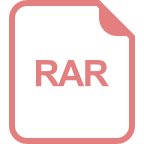





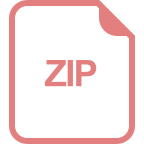
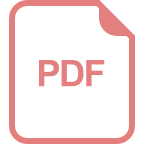
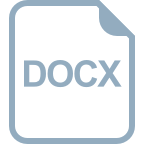
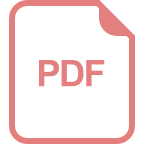
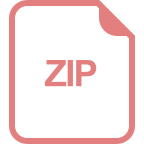
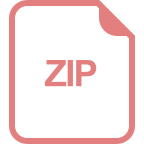
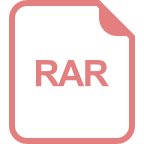
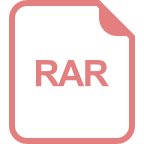

