代码实现支持qtableview操作支持撤销回退
时间: 2023-07-25 08:08:31 浏览: 42
要实现QTableView的撤销和恢复功能,需要使用QStandardItemModel和QUndoStack。首先,我们需要将QStandardItemModel设置为QTableView的模型。然后,我们可以创建QUndoStack对象并将其绑定到我们的QStandardItemModel上。最后,我们需要在用户编辑模型时创建一个QUndoCommand,并将其添加到QUndoStack中。这样,当用户撤销或恢复操作时,我们可以通过QUndoStack来恢复模型的状态。
下面是一个示例代码:
```python
from PyQt5.QtCore import Qt, QUndoCommand
from PyQt5.QtGui import QStandardItemModel
from PyQt5.QtWidgets import QTableView, QApplication, QMainWindow, QAction, QUndoStack
class TableModel(QStandardItemModel):
def __init__(self, parent=None):
super().__init__(parent)
self.undoStack = QUndoStack(self)
self.lastChangedIndex = None
def setData(self, index, value, role):
if role == Qt.EditRole:
oldData = self.data(index, role)
self.lastChangedIndex = index
command = EditCommand(self, index, value, oldData)
self.undoStack.push(command)
return super().setData(index, value, role)
class EditCommand(QUndoCommand):
def __init__(self, model, index, value, oldData):
super().__init__()
self.model = model
self.index = index
self.value = value
self.oldData = oldData
def undo(self):
self.model.setData(self.index, self.oldData, Qt.EditRole)
def redo(self):
self.model.setData(self.index, self.value, Qt.EditRole)
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.tableModel = TableModel(self)
self.tableView = QTableView(self)
self.tableView.setModel(self.tableModel)
self.setCentralWidget(self.tableView)
undoAction = QAction("撤销", self)
undoAction.setShortcut("Ctrl+Z")
undoAction.triggered.connect(self.tableModel.undoStack.undo)
redoAction = QAction("恢复", self)
redoAction.setShortcut("Ctrl+Shift+Z")
redoAction.triggered.connect(self.tableModel.undoStack.redo)
self.addAction(undoAction)
self.addAction(redoAction)
if __name__ == "__main__":
import sys
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个示例中,我们创建了一个TableModel类,它继承自QStandardItemModel。在TableModel中,我们创建了一个QUndoStack对象,并在setData方法中创建了一个EditCommand对象并将其添加到QUndoStack中。我们还保存了最后一个更改的索引,以便在撤销和恢复操作时使用。
在MainWindow中,我们创建了一个TableView并使用TableModel作为其模型。我们还创建了两个动作,用于撤销和恢复操作,并将其添加到窗口中。
当用户在TableView中编辑单元格时,我们会自动创建一个EditCommand,并将其添加到QUndoStack中。当用户执行撤销或恢复操作时,QUndoStack会自动调用EditCommand的undo或redo方法来恢复模型的状态。
相关推荐
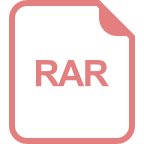
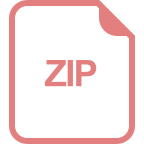
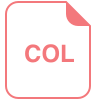
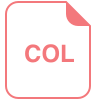
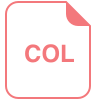
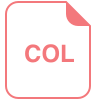










