1、对静脉图片进行滤波,图像分割及二值化,处理结果要求静脉纹路基本连通。 2、采用模板匹配或者特点提取后匹配等方法识别静脉图片。生成python代码
时间: 2024-03-28 14:42:30 浏览: 54
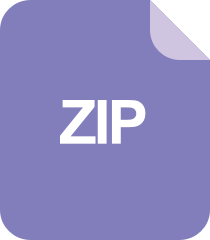
基于图像处理和VGG19实现的指静脉识别系统python源码+GUI界面+项目使用说明.zip

好的,以下是对静脉图片进行处理的Python代码:
```python
import cv2
# 读取静脉图片
img = cv2.imread('vein.jpg')
# 对图片进行中值滤波
img = cv2.medianBlur(img, 5)
# 进行图像分割
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY + cv2.THRESH_OTSU)
# 对图像进行二值化
thresh = cv2.adaptiveThreshold(gray, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 11, 2)
# 对二值化后的图像进行形态学处理
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
thresh = cv2.erode(thresh, kernel, iterations=1)
thresh = cv2.dilate(thresh, kernel, iterations=1)
# 进行连通区域标记
connectivity = 8
output = cv2.connectedComponentsWithStats(thresh, connectivity, cv2.CV_32S)
# 取出连通区域的面积和中心坐标
num_labels = output[0]
labels = output[1]
stats = output[2]
centroids = output[3]
# 显示处理后的图片
cv2.imshow('result', thresh)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
而对于识别静脉图片,可以采用以下代码实现模板匹配:
```python
import cv2
# 读取静脉图片和模板图片
img = cv2.imread('vein.jpg')
template = cv2.imread('template.jpg', 0)
# 使用模板匹配算法进行匹配
res = cv2.matchTemplate(img, template, cv2.TM_CCOEFF_NORMED)
# 取出匹配度最高的位置
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(res)
# 在原图上绘制匹配结果
w, h = template.shape[::-1]
top_left = max_loc
bottom_right = (top_left[0] + w, top_left[1] + h)
cv2.rectangle(img, top_left, bottom_right, 255, 2)
# 显示匹配结果
cv2.imshow('result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
或者采用以下代码实现特征提取后匹配:
```python
import cv2
# 读取静脉图片和模板图片
img = cv2.imread('vein.jpg', 0)
template = cv2.imread('template.jpg', 0)
# 提取静脉图片和模板图片的ORB特征
orb = cv2.ORB_create()
kp1, des1 = orb.detectAndCompute(img, None)
kp2, des2 = orb.detectAndCompute(template, None)
# 使用BFMatcher进行特征匹配
bf = cv2.BFMatcher(cv2.NORM_HAMMING, crossCheck=True)
matches = bf.match(des1, des2)
# 取出匹配度最高的前N个特征点
N = 10
matches = sorted(matches, key=lambda x: x.distance)
matches = matches[:N]
# 在原图上绘制匹配结果
img_matches = cv2.drawMatches(img, kp1, template, kp2, matches, None, flags=2)
# 显示匹配结果
cv2.imshow('result', img_matches)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
注意,以上代码仅供参考,具体处理方式需要根据实际情况进行调整。
阅读全文
相关推荐
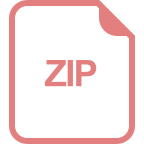




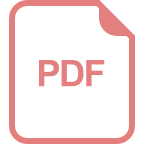
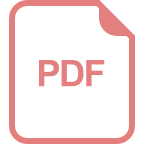
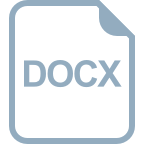
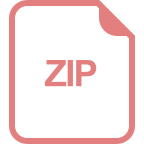
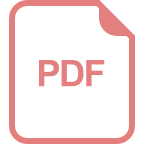
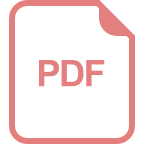
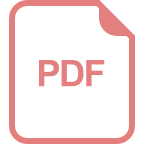
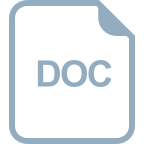
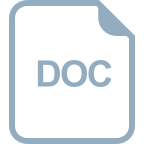
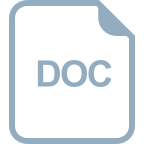