用C#开发CAD2016插件 将拾取的多段线向左缓冲1米构建region对象,如果有其它多段线与regio对象有重叠,则裁剪掉
时间: 2023-07-15 09:11:07 浏览: 42
开发CAD2016插件需要使用AutoCAD的API,具体步骤如下:
1. 创建新的C#类库项目,添加对`AcMgd.dll`和`AcDbMgd.dll`的引用。
2. 在类库中创建一个类,实现`IAcadCommand`接口,这个类将成为AutoCAD命令。
3. 在类中实现`Execute()`方法,用于处理命令逻辑。首先获取当前文档和编辑器对象:
```
Document doc = Application.DocumentManager.MdiActiveDocument;
Editor ed = doc.Editor;
```
4. 使用`PromptEntityOptions`和`PromptEntityResult`类获取用户选择的多段线对象:
```
PromptEntityOptions opt = new PromptEntityOptions("\n请选择一条多段线:");
opt.SetRejectMessage("\n只能选择多段线!");
opt.AddAllowedClass(typeof(Polyline), true);
PromptEntityResult res = ed.GetEntity(opt);
if (res.Status != PromptStatus.OK) return;
Polyline pline = null;
using (Transaction tr = doc.TransactionManager.StartTransaction())
{
pline = tr.GetObject(res.ObjectId, OpenMode.ForRead) as Polyline;
tr.Commit();
}
```
5. 构建区域对象:
```
Region region = null;
using (Transaction tr = doc.TransactionManager.StartTransaction())
{
DBObjectCollection objs = new DBObjectCollection();
Curve curve = null;
for (int i = 0; i < pline.NumberOfVertices - 1; i++)
{
Point3d startPt = pline.GetPoint3dAt(i);
Point3d endPt = pline.GetPoint3dAt(i + 1);
Line line = new Line(startPt, endPt);
curve = line.GetOffsetCurves(1)[0] as Curve;
objs.Add(curve);
}
region = Region.CreateFromCurves(objs);
tr.Commit();
}
```
6. 获取所有与区域对象相交的多段线,进行裁剪:
```
using (Transaction tr = doc.TransactionManager.StartTransaction())
{
BlockTable bt = tr.GetObject(doc.Database.BlockTableId, OpenMode.ForRead) as BlockTable;
BlockTableRecord btr = tr.GetObject(bt[BlockTableRecord.ModelSpace], OpenMode.ForWrite) as BlockTableRecord;
foreach (ObjectId id in btr)
{
Entity ent = tr.GetObject(id, OpenMode.ForRead) as Entity;
if (ent != null && ent.GetType() == typeof(Polyline))
{
Polyline poly = ent as Polyline;
if (poly.ObjectId != pline.ObjectId && region.IntersectWith(poly, Intersect.OnBothOperands, new Plane())[0] != null)
{
DBObjectCollection objs = new DBObjectCollection();
Curve curve = null;
for (int i = 0; i < poly.NumberOfVertices - 1; i++)
{
Point3d startPt = poly.GetPoint3dAt(i);
Point3d endPt = poly.GetPoint3dAt(i + 1);
Line line = new Line(startPt, endPt);
curve = line.GetOffsetCurves(1)[0] as Curve;
objs.Add(curve);
}
Region interRegion = Region.CreateFromCurves(objs);
if (region.Area > interRegion.Area)
{
region.BooleanOperation(BooleanOperationType.BoolIntersect, interRegion);
}
}
}
}
btr.AppendEntity(region);
tr.AddNewlyCreatedDBObject(region, true);
tr.Commit();
}
```
最后将类库编译生成dll文件,将其加载到AutoCAD中并输入命令名称即可使用。
相关推荐
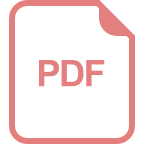
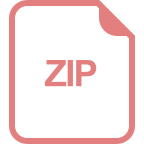
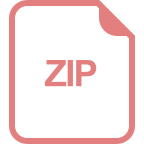



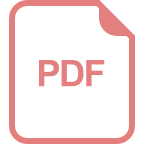
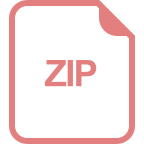
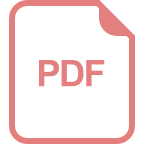
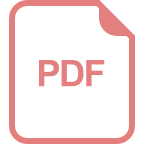
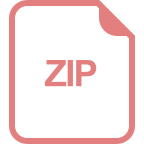
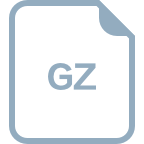
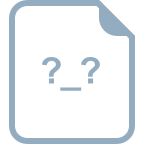
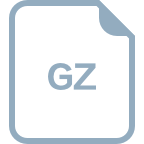