SpringBoot+vue做请求头中加token,请给写个详细完整的例子
时间: 2024-03-22 17:41:51 浏览: 15
好的,下面是一个Spring Boot和Vue.js的例子,用于在请求头中添加token。
1. 在Spring Boot中,您需要创建一个拦截器来处理请求头中的token。请参考以下代码:
```
@Component
public class JwtTokenInterceptor implements HandlerInterceptor {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
String token = request.getHeader("Authorization");
if (token != null && token.startsWith("Bearer ")) {
token = token.substring(7);
}
String username = jwtTokenUtil.getUsernameFromToken(token);
if (username != null && SecurityContextHolder.getContext().getAuthentication() == null) {
UserDetails userDetails = jwtTokenUtil.getUserDetailsFromToken(token);
if (jwtTokenUtil.validateToken(token, userDetails)) {
UsernamePasswordAuthenticationToken authenticationToken = new UsernamePasswordAuthenticationToken(userDetails, null, userDetails.getAuthorities());
authenticationToken.setDetails(new WebAuthenticationDetailsSource().buildDetails(request));
SecurityContextHolder.getContext().setAuthentication(authenticationToken);
}
}
return true;
}
}
```
这个拦截器将从请求头中获取token,并使用JwtTokenUtil类对其进行验证。如果token是有效的,则将其转换为Spring Security的身份验证对象,并将其添加到SecurityContextHolder中。
2. 在Spring Boot中,您还需要创建一个过滤器,用于在响应中添加token。请参考以下代码:
```
@Component
public class JwtTokenFilter extends OncePerRequestFilter {
@Autowired
private JwtTokenUtil jwtTokenUtil;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain chain) throws ServletException, IOException {
String token = jwtTokenUtil.generateToken(request.getUserPrincipal());
response.addHeader("Authorization", "Bearer " + token);
chain.doFilter(request, response);
}
}
```
这个过滤器将从Spring Security的身份验证对象中获取用户信息,并使用JwtTokenUtil类生成一个新的token。然后将该token添加到响应头中。
3. 在Vue.js中,您可以使用axios库来发送请求,并在请求头中添加token。请参考以下代码:
```
import axios from 'axios'
const api = axios.create({
baseURL: 'http://localhost:8080/api',
headers: {
'Content-Type': 'application/json'
}
})
api.interceptors.request.use(
config => {
const token = localStorage.getItem('token')
if (token) {
config.headers.Authorization = `Bearer ${token}`
}
return config
},
error => Promise.reject(error)
)
export default api
```
这个代码将创建一个名为“api”的axios实例,并将其基本URL设置为“http://localhost:8080/api”。然后,使用拦截器将请求头中的token添加到每个请求中。在这个例子中,token存储在localStorage中。
以上是用于在请求头中添加token的完整例子。请注意,在实际应用中,您需要根据您的需要对这些代码进行适当的修改和调整。
相关推荐
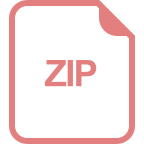
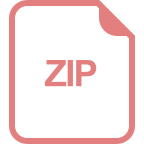
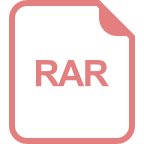














