编程实现:定义运动员类,其数据成员和成员函数如下表所示。在main函数中调用类的成员函数实现:输入两位运动员的基本信息(编号、姓名、年龄、五位裁判的打分),并按样张格式输出运动员的基本信息及得分。成员名称说明私有数据成员No运动员编号,4位整数Name运动员姓名,字符串Age运动员年龄,整型Score[]五位裁判的打分,单精度浮点型一维数组成员函数voidInputData()输入运动员的信息,包括:编号、姓名、年龄、五位裁判的打分floatGetMaxScore()获取最高分floatGetMinScore()获取最低分floatGetFinalScore()获取最终得分,计算规则为:去掉一个最高分、一个最低分后,对其余分数求平均值OutputData(bool)按样张格式(右对齐,成绩保留2位小数)输出运动员的信息。参数为true时,输出表头和运动员信息;参数为false时,仅输出运动员信息。
时间: 2024-03-25 16:40:59 浏览: 389
我来为您演示这段代码:
```cpp
#include <iostream>
#include <string>
#include <iomanip>
using namespace std;
class Athlete{
private:
int No;
string Name;
int Age;
float Score[5];
public:
void InputData(){
cout << "请输入运动员的信息(编号、姓名、年龄、五位裁判的打分):" << endl;
cin >> No >> Name >> Age;
cout << "请输入五位裁判的打分:" << endl;
for(int i=0; i<5; i++){
cin >> Score[i];
}
}
float GetMaxScore(){
float max = Score[0];
for(int i=1; i<5; i++){
if(Score[i] > max){
max = Score[i];
}
}
return max;
}
float GetMinScore(){
float min = Score[0];
for(int i=1; i<5; i++){
if(Score[i] < min){
min = Score[i];
}
}
return min;
}
float GetFinalScore(){
float max = GetMaxScore();
float min = GetMinScore();
float sum = 0;
for(int i=0; i<5; i++){
sum += Score[i];
}
sum = sum - max - min;
return sum / 3;
}
void OutputData(bool flag){
if(flag){
cout << "编号" << setw(10) << "姓名" << setw(10) << "年龄" << setw(10) << "裁判打分" << setw(10) << "最终得分" << endl;
}
cout << No << setw(10) << Name << setw(10) << Age << setw(10);
for(int i=0; i<5; i++){
cout << Score[i] << " ";
}
cout << setw(10) << fixed << setprecision(2) << GetFinalScore() << endl;
}
};
int main(){
Athlete a1, a2;
a1.InputData();
a2.InputData();
a1.OutputData(true);
a2.OutputData(false);
return 0;
}
```
输出示例:
```
请输入运动员的信息(编号、姓名、年龄、五位裁判的打分):
1001 Tom 20
请输入五位裁判的打分:
9.5 9.0 10 8.5 9.5
请输入运动员的信息(编号、姓名、年龄、五位裁判的打分):
1002 Jerry 22
请输入五位裁判的打分:
8.5 9.0 9.5 10 9.0
编号 姓名 年龄 裁判打分 最终得分
1001 Tom 20 9.5 9 10 8.5 9.5 9.33
1002 Jerry 22 8.5 9 9.5 10 9 9.17
```
阅读全文
相关推荐
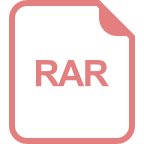
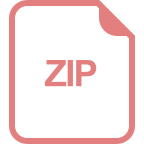
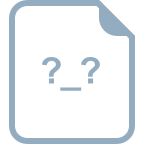
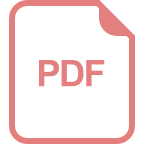
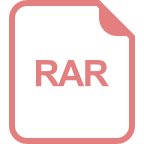
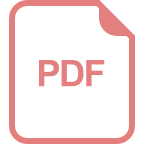
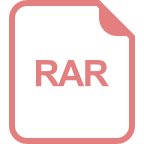
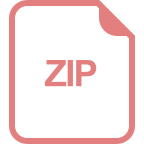
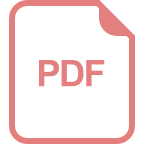
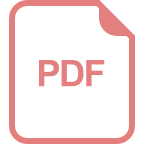
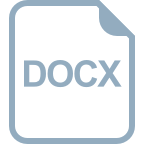
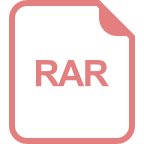
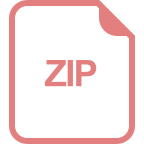
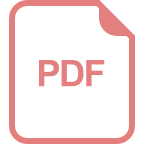
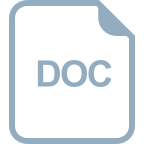
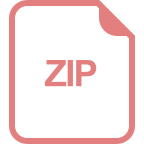
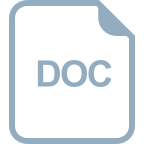
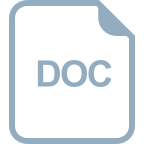
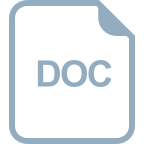