python tkinter 源码_matplotlib 与 tkinter 集成小案例 (上篇)
时间: 2024-05-07 22:23:36 浏览: 6
好的,以下是一个简单的例子,演示如何使用Matplotlib与Tkinter集成,实现一个简单的数据可视化应用程序。这个例子只是一个基础的应用程序,你可以根据自己的需求进行修改和扩展。
```python
import tkinter as tk
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
from matplotlib.figure import Figure
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
# 创建一个按钮
self.plot_button = tk.Button(self)
self.plot_button["text"] = "Plot Data"
self.plot_button["command"] = self.plot_data
self.plot_button.pack(side="top")
# 创建一个 Matplotlib 的 Figure 对象
self.fig = Figure(figsize=(5, 4), dpi=100)
# 在 Figure 对象中添加一个 Axes 对象
self.ax = self.fig.add_subplot(111)
# 在 Axes 对象中绘制一条曲线
self.x = [1, 2, 3, 4, 5]
self.y = [1, 4, 9, 16, 25]
self.ax.plot(self.x, self.y)
# 创建一个 Matplotlib 的画布,用于显示 Figure 对象
self.canvas = FigureCanvasTkAgg(self.fig, master=self)
self.canvas.draw()
self.canvas.get_tk_widget().pack(side="bottom", fill="both", expand=True)
def plot_data(self):
# 在 Axes 对象中绘制另一条曲线
self.ax.plot(self.x, [i ** 2 for i in self.x])
self.canvas.draw()
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
这个例子中,我们首先创建了一个继承自`tk.Frame`的类`Application`,然后在类的构造函数中创建了一个按钮和一个Matplotlib的Figure对象。我们在Figure对象中创建了一个Axes对象,并在Axes对象中绘制了一条曲线。接着,我们创建了一个Matplotlib的画布,并将Figure对象显示在画布上。
当用户点击按钮时,我们在Axes对象中绘制了另一条曲线,并通过调用`canvas.draw()`方法,将更新后的Figure对象显示在画布上。
你可以尝试运行这个例子,看看它是如何工作的。
相关推荐
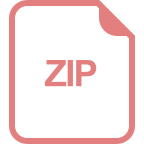
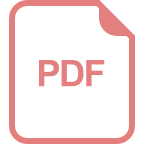
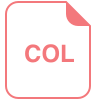
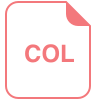
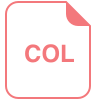
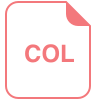
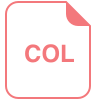









