多线条共用x轴,python代码
时间: 2023-04-11 19:01:38 浏览: 129
你好,我可以回答这个问题。在Python中,可以使用matplotlib库中的subplot函数来实现多线条共用x轴的效果。具体实现方法可以参考以下代码:
```
import matplotlib.pyplot as plt
# 创建一个figure对象
fig = plt.figure()
# 创建两个子图,共用x轴
ax1 = fig.add_subplot(2, 1, 1)
ax2 = fig.add_subplot(2, 1, 2, sharex=ax1)
# 绘制两条线条
ax1.plot([1, 2, 3], [4, 5, 6])
ax2.plot([1, 2, 3], [2, 4, 6])
# 显示图像
plt.show()
```
这段代码中,我们首先创建了一个figure对象,然后使用add_subplot函数创建了两个子图,其中第二个子图使用了sharex参数来共用第一个子图的x轴。最后,我们在两个子图中分别绘制了一条线条,并使用plt.show()函数显示图像。
相关问题
matplotlib多线条共用x轴
可以使用plt.subplots()函数创建多个子图,每个子图都共用同一个x轴,然后在每个子图中分别绘制不同的线条。具体代码如下:
```python
import matplotlib.pyplot as plt
# 创建两个子图,共用同一个x轴
fig, ax = plt.subplots(2, 1, sharex=True)
# 在第一个子图中绘制线条1
ax[0].plot([1, 2, 3], [4, 5, 6], label='Line 1')
# 在第二个子图中绘制线条2
ax[1].plot([1, 2, 3], [2, 4, 6], label='Line 2')
# 添加图例和标签
ax[0].legend()
ax[1].legend()
ax[1].set_xlabel('X Label')
ax[0].set_ylabel('Y Label 1')
ax[1].set_ylabel('Y Label 2')
# 显示图形
plt.show()
```
这段代码会创建两个子图,分别绘制线条1和线条2,两个子图共用同一个x轴。最后添加图例和标签,并显示图形。
matlab共用x轴
在 MATLAB 中,可以通过使用 subplot 函数来实现共用 x 轴的功能。下面是一个示例代码:
```matlab
% 创建两个子图
subplot(2,1,1);
% 绘制第一个子图的数据
x1 = 0:0.1:10;
y1 = sin(x1);
plot(x1, y1);
title('第一个子图');
subplot(2,1,2);
% 绘制第二个子图的数据
x2 = 0:0.5:10;
y2 = cos(x2);
plot(x2, y2);
title('第二个子图');
% 设置共用 x 轴
linkaxes([subplot(2,1,1), subplot(2,1,2)], 'x');
```
在上面的示例中,我们创建了两个子图,并分别绘制了不同的数据。然后,通过 `linkaxes` 函数将两个子图的 x 轴链接起来,实现共用 x 轴的效果。
相关推荐
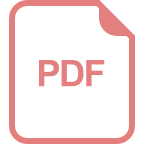
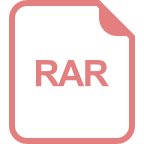












