rust +=重载操作符
时间: 2023-08-03 09:42:59 浏览: 167
在 Rust 中,可以通过实现 `std::ops::AddAssign` trait 来重载 `+=` 操作符。这个 trait 定义了一个 `add_assign` 方法,用于将另一个值加到当前值上。下面是一个示例:
```rust
use std::ops::AddAssign;
struct MyNumber(i32);
impl AddAssign for MyNumber {
fn add_assign(&mut self, other: Self) {
self.0 += other.0;
}
}
fn main() {
let mut x = MyNumber(5);
x += MyNumber(3);
println!("{}", x.0); // 输出 8
}
```
在上面的例子中,我们定义了一个名为 `MyNumber` 的结构体,并实现了 `AddAssign` trait。在 `main` 函数中,我们可以使用 `+=` 操作符将一个 `MyNumber` 值加到另一个 `MyNumber` 值上,并打印结果。
相关问题
rust 多重 操作符重载方法 以及 被加数为标量 或者向量
在 Rust 中,可以使用 trait 来重载操作符。可以通过实现特定 trait 来重载多种操作符,包括加法操作符(Add trait)、减法操作符(Sub trait)、乘法操作符(Mul trait)和除法操作符(Div trait)等等。
下面是一个例子,展示了如何重载加法操作符(Add trait):
```rust
use std::ops::Add;
// 定义一个标量结构体
struct Scalar {
value: f32,
}
// 实现标量结构体的 Add trait
impl Add<Scalar> for Scalar {
type Output = Scalar;
fn add(self, other: Scalar) -> Scalar {
Scalar { value: self.value + other.value }
}
}
// 定义一个向量结构体
struct Vector {
x: f32,
y: f32,
z: f32,
}
// 实现向量结构体的 Add trait
impl Add<Vector> for Vector {
type Output = Vector;
fn add(self, other: Vector) -> Vector {
Vector { x: self.x + other.x, y: self.y + other.y, z: self.z + other.z }
}
}
// 测试
fn main() {
let a = Scalar { value: 1.0 };
let b = Scalar { value: 2.0 };
let c = a + b;
println!("{}", c.value);
let d = Vector { x: 1.0, y: 2.0, z: 3.0 };
let e = Vector { x: 4.0, y: 5.0, z: 6.0 };
let f = d + e;
println!("({}, {}, {})", f.x, f.y, f.z);
}
```
在上面的例子中,我们定义了一个标量结构体 `Scalar` 和一个向量结构体 `Vector`,并分别实现了它们的 `Add` trait。在实现 `Add` trait 时,需要指定输出类型 `type Output = Scalar` 或 `type Output = Vector`,并实现 `add` 方法来执行加法操作。在 `add` 方法中,我们创建了一个新的 `Scalar` 或 `Vector` 实例,其值为两个操作数的和。
如果我们想要重载被加数为标量或者向量的加法操作符,可以分别实现 `Add<Scalar>` 和 `Add<Vector>` trait。例如:
```rust
use std::ops::Add;
// 定义标量和向量之间的加法操作
impl Add<Scalar> for Vector {
type Output = Vector;
fn add(self, other: Scalar) -> Vector {
Vector { x: self.x + other.value, y: self.y + other.value, z: self.z + other.value }
}
}
impl Add<Vector> for Scalar {
type Output = Vector;
fn add(self, other: Vector) -> Vector {
Vector { x: self.value + other.x, y: self.value + other.y, z: self.value + other.z }
}
}
// 测试
fn main() {
let a = Scalar { value: 1.0 };
let b = Vector { x: 2.0, y: 3.0, z: 4.0 };
let c = b + a;
println!("({}, {}, {})", c.x, c.y, c.z);
let d = Vector { x: 1.0, y: 2.0, z: 3.0 };
let e = Scalar { value: 2.0 };
let f = e + d;
println!("({}, {}, {})", f.x, f.y, f.z);
}
```
在上面的例子中,我们分别实现了 `Add<Scalar>` 和 `Add<Vector>` trait,实现了标量和向量之间的加法操作。在 `Add<Scalar>` 中,我们重载了 `Vector` 和 `Scalar` 的加法操作符,将标量的值加到向量的每个分量上,最后返回一个新的 `Vector` 实例;在 `Add<Vector>` 中,我们重载了 `Scalar` 和 `Vector` 的加法操作符,将标量的值加到向量的每个分量上,最后返回一个新的 `Vector` 实例。
阅读全文
相关推荐
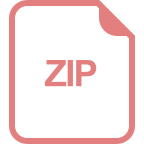
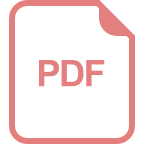
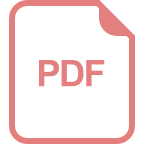
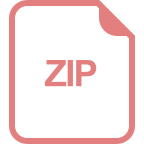
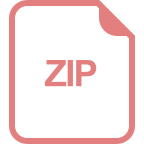
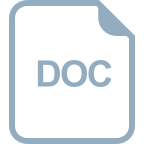
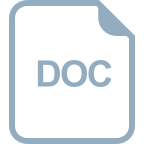
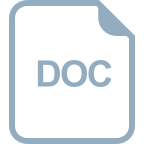
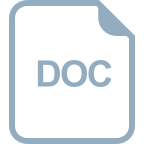
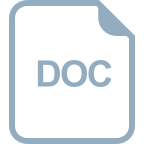
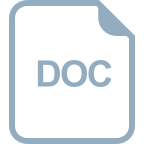
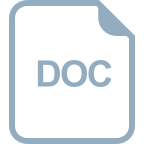
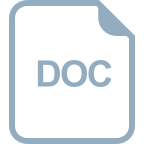
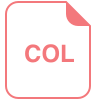
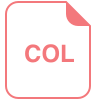