JAVA中MVC写BMI
时间: 2023-07-16 20:13:42 浏览: 50
MVC(Model-View-Controller)是一种设计模式,用于将应用程序的不同方面分离开来,以便更好地进行维护和扩展。在Java中,可以使用MVC模式来编写BMI计算器。
1. Model(模型):负责计算BMI值。
```java
public class BMICalculator {
private double height;
private double weight;
public void setHeight(double height) {
this.height = height;
}
public void setWeight(double weight) {
this.weight = weight;
}
public double calculateBMI() {
return weight / (height * height);
}
}
```
2. View(视图):负责显示界面和接收用户输入。
```java
import java.util.Scanner;
public class BMIView {
private Scanner scanner;
public BMIView() {
scanner = new Scanner(System.in);
}
public void displayBMI(double bmi) {
System.out.println("Your BMI is " + bmi);
}
public double getHeight() {
System.out.print("Enter your height in meters: ");
return scanner.nextDouble();
}
public double getWeight() {
System.out.print("Enter your weight in kilograms: ");
return scanner.nextDouble();
}
}
```
3. Controller(控制器):负责协调Model和View。
```java
public class BMIController {
private BMICalculator model;
private BMIView view;
public BMIController(BMICalculator model, BMIView view) {
this.model = model;
this.view = view;
}
public void calculateBMI() {
double height = view.getHeight();
double weight = view.getWeight();
model.setHeight(height);
model.setWeight(weight);
double bmi = model.calculateBMI();
view.displayBMI(bmi);
}
}
```
在主函数中,创建Model、View和Controller,并调用Controller的calculateBMI方法。
```java
public class Main {
public static void main(String[] args) {
BMICalculator model = new BMICalculator();
BMIView view = new BMIView();
BMIController controller = new BMIController(model, view);
controller.calculateBMI();
}
}
```
相关推荐
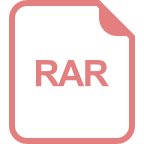
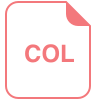
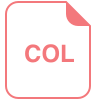
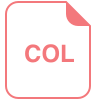
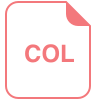
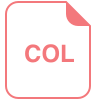









