如何用C++代码实现这个计算过程?
时间: 2024-09-14 08:06:00 浏览: 33
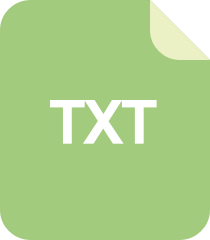
基于c++代码实现的三次样条插值
在C++中,你可以创建一个函数来解决这个问题。首先,你需要两个变量分别代表鸡和兔子的数量,以及一个额外的变量存储总腿数。这里是一个简单的示例:
```cpp
#include <iostream>
int chickenLegs(int m, int n) {
return 2 * m;
}
int rabbitLegs(int m, int n) {
return 4 * n;
}
int totalLegs(int m, int n) {
return chickenLegs(m, n) + rabbitLegs(m, n);
}
std::pair<int, int> countChickensAnd Rabbits(int legs) {
for (int i = 0; i <= m + n; ++i) { // 推测范围,因为鸡兔总数不能超过m+n
int rabbits = i;
int chickens = m + n - rabbits;
if (totalLegs(chickens, rabbits) == legs) {
return std::make_pair(chickens, rabbits);
}
}
throw std::runtime_error("No solution found for the given leg count.");
}
int main() {
int m, n, totalLegsGiven;
std::cout << "Enter the number of chickens (m): ";
std::cin >> m;
std::cout << "Enter the number of rabbits (n): ";
std::cin >> n;
totalLegsGiven = totalLegs(m, n);
std::cout << "Total legs: " << totalLegsGiven << std::endl;
try {
auto result = countChickensAndRabbits(totalLegsGiven);
std::cout << "For " << totalLegsGiven << " legs, you have " << result.first << " chickens and " << result.second << " rabbits." << std::endl;
} catch (const std::exception &e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
```
在这个代码中,`countChickensAndRabbits` 函数会尝试找出所有可能的鸡兔组合,使得它们的腿数匹配给定的值。注意,这个算法可能不是最优的,特别是当腿的数量非常大时,因为它采用了穷举法。
阅读全文
相关推荐
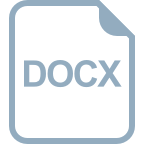
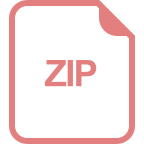
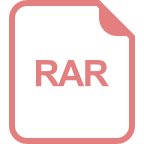
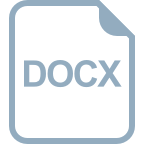
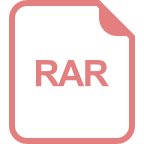
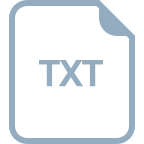
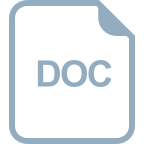
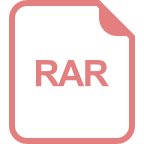
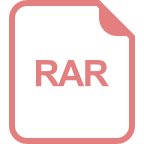
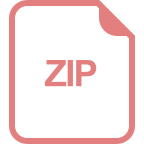
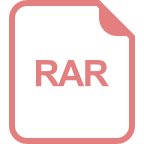
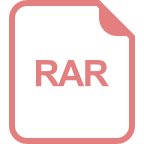
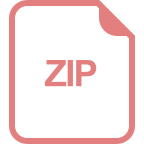
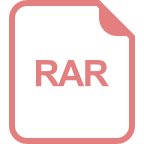
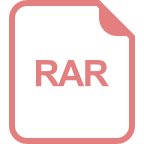
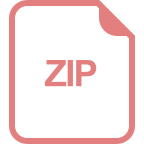
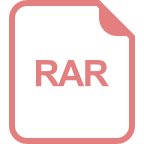
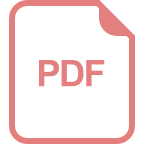