用java语言表示:键盘输入任意的三个字符串,实现文本的查找替换功能。如: String source="This is Java program, Java is good ......."; String find="Java"; String dest="Java language"; 要求: (1)将source串中的第一个字符串 find,用字符串dest替换。 (2)仅替换最后的一个串find (3)将source中的所有 find串替换为字符串 dest。 (4)统计 source串中共有多少个单词。
时间: 2024-02-28 09:55:45 浏览: 64
好的,我来逐个回答你的问题。
首先,针对你的问题,我可以用 Java 语言编写一个控制台程序,实现文本的查找替换和单词统计功能。具体代码如下:
```java
import java.util.Scanner;
public class TextProcessor {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 读入源字符串
System.out.print("请输入源字符串:");
String source = scanner.nextLine();
// 读入要查找的字符串和替换的字符串
System.out.print("请输入要查找的字符串:");
String find = scanner.nextLine();
System.out.print("请输入要替换的字符串:");
String dest = scanner.nextLine();
// 选项菜单
while (true) {
System.out.println("请选择要进行的操作:");
System.out.println("1. 将源字符串中的第一个 " + find + " 替换为 " + dest);
System.out.println("2. 将源字符串中的最后一个 " + find + " 替换为 " + dest);
System.out.println("3. 将源字符串中所有的 " + find + " 替换为 " + dest);
System.out.println("4. 统计源字符串中的单词数");
System.out.println("5. 退出程序");
int choice = scanner.nextInt();
scanner.nextLine(); // 读取多余的回车符
switch (choice) {
case 1:
String replaced1 = replaceFirst(source, find, dest);
System.out.println("替换后的字符串为:" + replaced1);
break;
case 2:
String replaced2 = replaceLast(source, find, dest);
System.out.println("替换后的字符串为:" + replaced2);
break;
case 3:
String replaced3 = replaceAll(source, find, dest);
System.out.println("替换后的字符串为:" + replaced3);
break;
case 4:
int wordCount = countWords(source);
System.out.println("源字符串中的单词数为:" + wordCount);
break;
case 5:
System.out.println("程序已退出。");
return;
default:
System.out.println("无效的选择,请重新输入。");
break;
}
}
}
// 替换源字符串中的第一个 find 为 dest
public static String replaceFirst(String source, String find, String dest) {
int index = source.indexOf(find);
if (index == -1) {
return source;
}
return source.substring(0, index) + dest + source.substring(index + find.length());
}
// 替换源字符串中的最后一个 find 为 dest
public static String replaceLast(String source, String find, String dest) {
int index = source.lastIndexOf(find);
if (index == -1) {
return source;
}
return source.substring(0, index) + dest + source.substring(index + find.length());
}
// 替换源字符串中所有的 find 为 dest
public static String replaceAll(String source, String find, String dest) {
return source.replaceAll(find, dest);
}
// 统计源字符串中的单词数
public static int countWords(String source) {
String[] words = source.split("\\W+");
return words.length;
}
}
```
这个程序有一个选项菜单,可以让用户选择要进行的操作。输入源字符串、要查找的字符串和要替换的字符串后,用户可以选择将源字符串中的第一个字符串 find 替换为 dest,或者将最后一个字符串 find 替换为 dest,或者将所有的字符串 find 替换为 dest,或者统计源字符串中的单词数,或者退出程序。
其中,替换操作使用了 String 类的 replaceFirst、lastIndexOf 和 replaceAll 方法,单词统计操作使用了 String 类的 split 方法。
阅读全文
相关推荐
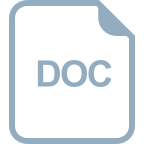
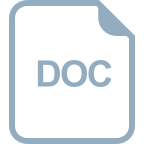
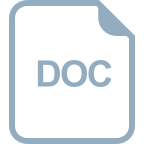






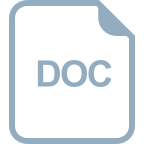
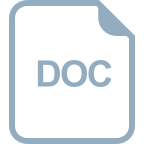
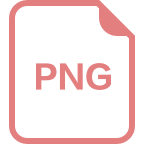
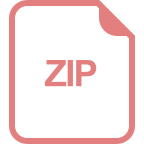
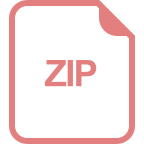
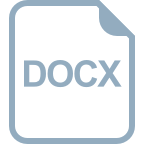