我的评论数据第一列是评论,第二列是情感分类,分为positive、negative、neutral三种情感,给我一个完整的情感三分类的svm代码,告诉我模型是怎么训练的,对了,你最后把这三种情感的roc曲线画到一张图里面
时间: 2024-02-25 11:58:30 浏览: 22
好的,以下是一个完整的情感三分类的SVM代码,包括数据的读取和处理、模型的训练和预测、以及ROC曲线的绘制:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.svm import SVC
from sklearn.metrics import roc_curve, auc
# 读取数据
data = pd.read_csv('your_data.csv')
# 将情感分类转换为数值
data['sentiment'] = data['sentiment'].map({'positive': 1, 'negative': -1, 'neutral': 0})
# 分离特征和标签
X = data['comment'].values
y = data['sentiment'].values
# 将文本特征转换为数值特征
from sklearn.feature_extraction.text import TfidfVectorizer
vectorizer = TfidfVectorizer(max_features=10000) # 只取前10000个特征
X = vectorizer.fit_transform(X)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练SVM模型
svm = SVC(kernel='linear', probability=True)
svm.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = svm.predict(X_test)
# 计算ROC曲线和AUC值
fpr, tpr, thresholds = roc_curve(y_test, y_pred, pos_label=1)
roc_auc = auc(fpr, tpr)
# 绘制ROC曲线
plt.plot(fpr, tpr, color='darkorange', lw=2, label='ROC curve (area = %0.2f)' % roc_auc)
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
```
这段代码首先读取了包含评论和情感分类的CSV文件,并将情感分类转换为数值。然后将文本特征转换为数值特征,并划分训练集和测试集。接着使用SVM模型进行训练和预测,并计算ROC曲线和AUC值。最后使用Matplotlib库绘制ROC曲线。
请注意,这里使用了TF-IDF算法将文本特征转换为数值特征,因为SVM模型只能处理数值特征。同时,为了避免特征过于稀疏,只取了前10000个特征。您可以根据实际数据的特点调整这些参数。
另外,为了让ROC曲线更加直观,可以将三种情感的数据分别绘制在同一张图里面。您可以使用以下代码实现:
```python
# 分别计算三种情感的ROC曲线和AUC值
fpr_pos, tpr_pos, thresholds_pos = roc_curve(y_test[y_test == 1], y_pred[y_test == 1], pos_label=1)
roc_auc_pos = auc(fpr_pos, tpr_pos)
fpr_neg, tpr_neg, thresholds_neg = roc_curve(y_test[y_test == -1], y_pred[y_test == -1], pos_label=-1)
roc_auc_neg = auc(fpr_neg, tpr_neg)
fpr_neu, tpr_neu, thresholds_neu = roc_curve(y_test[y_test == 0], y_pred[y_test == 0], pos_label=0)
roc_auc_neu = auc(fpr_neu, tpr_neu)
# 绘制ROC曲线
plt.plot(fpr_pos, tpr_pos, color='darkorange', lw=2, label='Positive (area = %0.2f)' % roc_auc_pos)
plt.plot(fpr_neg, tpr_neg, color='blue', lw=2, label='Negative (area = %0.2f)' % roc_auc_neg)
plt.plot(fpr_neu, tpr_neu, color='green', lw=2, label='Neutral (area = %0.2f)' % roc_auc_neu)
plt.plot([0, 1], [0, 1], color='navy', lw=2, linestyle='--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('Receiver operating characteristic')
plt.legend(loc="lower right")
plt.show()
```
这段代码首先分别计算了三种情感的ROC曲线和AUC值,然后将它们分别绘制在同一张图里面。
相关推荐
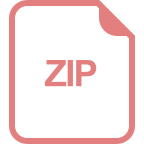
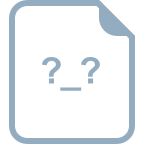














