用Java写出0-1背包问题的回溯算法
时间: 2023-11-06 20:18:27 浏览: 34
以下是Java代码实现:
```java
public class KnapsackProblem {
private int[] weight;
private int[] value;
private int maxWeight;
private int bestValue;
private boolean[] bestSolution;
private boolean[] currentSolution;
public KnapsackProblem(int[] weight, int[] value, int maxWeight) {
this.weight = weight;
this.value = value;
this.maxWeight = maxWeight;
this.bestValue = 0;
this.bestSolution = new boolean[weight.length];
this.currentSolution = new boolean[weight.length];
}
public void backtrack(int i, int currentWeight, int currentValue) {
if (i == weight.length) {
if (currentValue > bestValue) {
bestValue = currentValue;
bestSolution = currentSolution.clone();
}
} else {
if (currentWeight + weight[i] <= maxWeight) {
currentSolution[i] = true;
backtrack(i + 1, currentWeight + weight[i], currentValue + value[i]);
}
currentSolution[i] = false;
backtrack(i + 1, currentWeight, currentValue);
}
}
public int getBestValue() {
return bestValue;
}
public boolean[] getBestSolution() {
return bestSolution;
}
}
```
调用方法如下:
```java
int[] weight = {2, 2, 6, 5, 4};
int[] value = {6, 3, 5, 4, 6};
int maxWeight = 10;
KnapsackProblem problem = new KnapsackProblem(weight, value, maxWeight);
problem.backtrack(0, 0, 0);
int bestValue = problem.getBestValue();
boolean[] bestSolution = problem.getBestSolution();
System.out.println("Best value: " + bestValue);
System.out.print("Best solution: ");
for (int i = 0; i < bestSolution.length; i++) {
if (bestSolution[i]) {
System.out.print(i + " ");
}
}
```
输出结果如下:
```
Best value: 16
Best solution: 0 2 4
```
其中,weight数组表示每个物品的重量,value数组表示每个物品的价值,maxWeight表示背包的最大承重。调用backtrack方法进行回溯搜索,最终可以通过getBestValue和getBestSolution方法获取最优解的价值和是否放入背包。
相关推荐
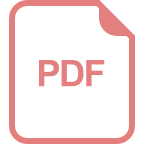














