Python程序来绘制皮卡丘
时间: 2023-11-05 16:17:26 浏览: 106
好的,让我们来画一个皮卡丘!
首先,我们需要导入一些库:
```python
import turtle
import math
```
然后,我们可以设置一些画笔的属性:
```python
turtle.penup()
turtle.speed(10)
turtle.bgcolor("#ffde00")
turtle.color("#000000")
turtle.pensize(3)
```
接下来,我们可以定义一些函数,帮助我们绘制皮卡丘:
```python
# 画圆函数
def draw_circle(x, y, r, color):
turtle.penup()
turtle.goto(x, y-r)
turtle.pendown()
turtle.fillcolor(color)
turtle.begin_fill()
turtle.circle(r)
turtle.end_fill()
# 画半圆函数
def draw_semicircle(x, y, r, color):
turtle.penup()
turtle.goto(x, y-r)
turtle.pendown()
turtle.fillcolor(color)
turtle.begin_fill()
turtle.circle(r, 180)
turtle.end_fill()
# 画眼睛函数
def draw_eyes(x, y, r, color):
draw_circle(x, y, r, color)
draw_circle(x, y, r*0.5, "#ffffff")
draw_circle(x, y, r*0.2, "#000000")
# 画腮红函数
def draw_cheeks(x, y, r, color):
draw_circle(x, y, r, color)
# 画嘴巴函数
def draw_mouth(x, y, r, color):
turtle.penup()
turtle.goto(x-r, y)
turtle.pendown()
turtle.color(color)
turtle.circle(r, 180)
# 画尖角函数
def draw_triangle(x, y, size, color):
turtle.penup()
turtle.goto(x, y)
turtle.pendown()
turtle.fillcolor(color)
turtle.begin_fill()
for i in range(3):
turtle.forward(size)
turtle.left(120)
turtle.end_fill()
# 画耳朵函数
def draw_ears(x, y, r, color):
draw_semicircle(x, y, r, color)
draw_triangle(x, y+r*0.1, r*0.4, color)
# 画身体函数
def draw_body(x, y, w, h, color):
turtle.penup()
turtle.goto(x-w/2, y-h/2)
turtle.pendown()
turtle.fillcolor(color)
turtle.begin_fill()
for i in range(2):
turtle.forward(w)
turtle.left(90)
turtle.forward(h)
turtle.left(90)
turtle.end_fill()
```
最后,我们可以调用这些函数来绘制皮卡丘:
```python
# 画耳朵
draw_ears(-80, 160, 50, "#f8bbd0")
draw_ears(80, 160, 50, "#f8bbd0")
# 画头部和脸部
draw_circle(0, 0, 150, "#f8bbd0")
draw_circle(0, 30, 120, "#ffffff")
# 画眼睛
draw_eyes(-45, 70, 30, "#000000")
draw_eyes(45, 70, 30, "#000000")
# 画腮红
draw_cheeks(-80, -20, 30, "#ff9e9e")
draw_cheeks(80, -20, 30, "#ff9e9e")
# 画嘴巴
draw_mouth(0, -20, 70, "#000000")
# 画身体
draw_body(0, -250, 250, 300, "#f8bbd0")
```
完成了!现在你可以看到一个可爱的皮卡丘画在了屏幕上。
阅读全文
相关推荐
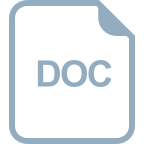
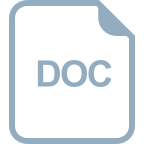
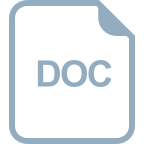

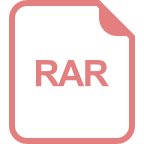
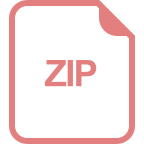
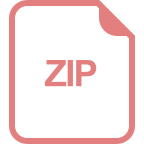
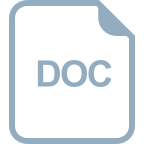
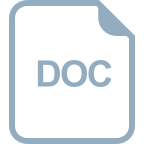








