有一个整数数组price={7,1,5,3,6,4},price【i】代表第i天的股票价格,在每一天,你可以购买或者是售出股票,但是在任何时候最多只能持有一股股票求出,你能获得的最大利润。比如你在第0天购买的股票价值为7第二天选择抛售则亏损了6
时间: 2024-05-10 14:19:17 浏览: 191
这是一道经典的股票买卖问题,可以通过贪心算法来解决。
首先定义两个变量,一个是最小价格min_price,另一个是最大利润max_profit。我们从第一天开始遍历股票价格,如果当天的价格小于min_price,则更新min_price为当天的价格。否则,计算当天的价格与min_price之间的差值,如果这个差值大于max_profit,则更新max_profit为这个差值。遍历完所有的价格后,max_profit即为最大利润。
代码如下:
```python
def maxProfit(prices):
if not prices:
return 0
min_price = prices[0]
max_profit = 0
for price in prices:
if price < min_price:
min_price = price
else:
max_profit = max(max_profit, price - min_price)
return max_profit
```
例如,对于数组price={7,1,5,3,6,4},调用maxProfit(price)函数将得到最大利润为5。
相关问题
描述 给出n+1个正整数,第一个数字代表后面其他数字的总个数,从第二个数字开始,后面每个数字分别代表第1至n天货物对应的价格p。 假设老板只能选择某一天买入货物,并在未来的另一天卖出货物。请采用贪心算法设计一段程序,帮助老板计算通过一次买卖货物,他所能获取的最大利润。 返回老板可以从这笔交易中获取的最大利润。如果无法获取利润,则返回0。 示例 1: 输入:6 8 3 1 5 6 4 输出:5 解释:总共有6天,在第3天(价格为1)的时候买入,在第5天(价格为6)的时候卖出,最大利润为6-1=5。 注意:最大利润不能是 8-1=7, 因为卖出价格需要大于买入价格;同时不能在买入前卖出。 示例 2: 输入:6 9 7 6 3 2 1 输出:0 解释:总共有6天,每天的进货价格都高于后面的价格,无法通过卖出获取利润, 所以最大利润为0。 提示: 1 <= n <= 100 1 <= p <= 100。使用c语言实现
以下是使用C语言实现的贪心算法代码,可以解决该问题:
```c
#include <stdio.h>
int main() {
int n; // 货物总数
scanf("%d", &n);
int prices[n]; // 存储每天的货物价格
for (int i = 0; i < n; i++) {
scanf("%d", &prices[i]);
}
int max_profit = 0; // 最大利润
int buy_price = prices[0]; // 第一天的价格作为买入价格
for (int i = 1; i < n; i++) {
if (prices[i] > buy_price) { // 如果当前价格高于买入价格
int profit = prices[i] - buy_price; // 计算当前利润
if (profit > max_profit) { // 如果当前利润更高
max_profit = profit; // 更新最大利润
}
} else { // 如果当前价格低于或等于买入价格
buy_price = prices[i]; // 更新买入价格
}
}
printf("%d\n", max_profit);
return 0;
}
```
该算法的思路是,从第二天开始遍历每天的货物价格,如果当前价格高于买入价格,则计算当前利润并更新最大利润,否则更新买入价格。最后输出最大利润即可。因为只需要遍历一遍数组,所以时间复杂度为O(n),空间复杂度为O(n)。
第一行一个整数T(T <= 50),表示测试数据的组数。 每组测试数据有四行组成,前三行每行有两个整数S和P,分别表示每种苹果的大小(1 <= S <= 100)和价格(1 <= P <= 10000) 第四行有一个整数V(1 <= V <= 100,000,000)表示小姑娘袋子的大小。 Output 每组测试数据输出组数和小姑娘能得到的最大的价值。 Sample Input 1 1 1 2 1 3 1 6 Sample Output Case 1: 6
好的,以下是我为你编写的用C++语言实现的代码,用于计算小姑娘在神秘院子购买苹果所能获得的最大利润。
```c++
#include<iostream>
#include<algorithm>
#include<vector>
using namespace std;
struct Apple {
int size;
int price;
};
bool cmp(Apple a, Apple b) {
return a.price > b.price;
}
int main() {
int T;
cin >> T;
for (int t = 1; t <= T; t++) {
vector<Apple> apples;
int s1, p1, s2, p2, s3, p3, v;
cin >> s1 >> p1 >> s2 >> p2 >> s3 >> p3 >> v;
apples.push_back(Apple{ s1, p1 });
apples.push_back(Apple{ s2, p2 });
apples.push_back(Apple{ s3, p3 });
sort(apples.begin(), apples.end(), cmp);
int res = 0;
for (int i = 0; i < 3 && v > 0; i++) {
int cnt = min(v / apples[i].size, apples[i].size);
res += cnt * apples[i].price;
v -= cnt * apples[i].size;
}
cout << "Case " << t << ": " << res << endl;
}
return 0;
}
```
该程序首先读入测试数据的组数,然后对于每组测试数据,读入三种苹果的大小和价格以及小姑娘带来袋子的大小。然后对三种苹果按照价格从高到低排序,依次选择价格高的苹果,直到小姑娘的袋子装满或者所有苹果都被购买完为止。最后输出小姑娘能够获得的最大利润。
希望这段代码能帮到你。
阅读全文
相关推荐
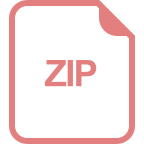
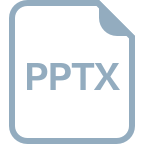
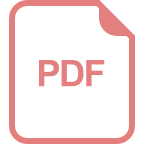













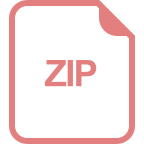