mybatis,将数组入参转化为临时表,可以join操作
时间: 2023-12-06 21:44:26 浏览: 202
在 MyBatis 中,我们可以使用自定义类型处理器将数组入参转换为临时表,并使用该临时表进行 join 操作。下面是一些大致的步骤:
1. 创建一个自定义类型处理器,实现 TypeHandler 接口,并在其中编写将数组转换为临时表的逻辑。
2. 在 MyBatis 的配置文件中配置该自定义类型处理器。
3. 在 Mapper 接口中编写 SQL 语句,使用该自定义类型处理器将数组入参转换为临时表,并进行 join 操作。
以下是一些示例代码,帮助你更好地理解:
1. 自定义类型处理器
```java
public class ArrayTypeHandler implements TypeHandler<Object[]> {
@Override
public void setParameter(PreparedStatement ps, int i, Object[] parameter, JdbcType jdbcType) throws SQLException {
// 将数组转换为临时表
Connection connection = ps.getConnection();
String tempTableName = "temp_table_" + UUID.randomUUID().toString().replace("-", "");
PreparedStatement createTempTableStatement = connection.prepareStatement("CREATE TEMPORARY TABLE " + tempTableName + " (id INT)");
createTempTableStatement.executeUpdate();
createTempTableStatement.close();
PreparedStatement insertTempTableStatement = connection.prepareStatement("INSERT INTO " + tempTableName + " VALUES (?)");
for (Object item : parameter) {
insertTempTableStatement.setObject(1, item);
insertTempTableStatement.addBatch();
}
insertTempTableStatement.executeBatch();
insertTempTableStatement.close();
// 设置参数为临时表
ps.setString(i, tempTableName);
}
@Override
public Object[] getResult(ResultSet rs, String columnName) throws SQLException {
throw new UnsupportedOperationException("Not implemented");
}
@Override
public Object[] getResult(ResultSet rs, int columnIndex) throws SQLException {
throw new UnsupportedOperationException("Not implemented");
}
@Override
public Object[] getResult(CallableStatement cs, int columnIndex) throws SQLException {
throw new UnsupportedOperationException("Not implemented");
}
}
```
2. MyBatis 配置文件中配置自定义类型处理器
```xml
<typeHandlers>
<typeHandler handler="com.example.ArrayTypeHandler"/>
</typeHandlers>
```
3. Mapper 接口中编写 SQL 语句
```xml
<select id="getUsersByIDs" resultMap="userMap">
SELECT u.id, u.name, u.age, r.role_name
FROM users u
JOIN roles r ON u.role_id = r.id
JOIN ${ids, typeHandler=com.example.ArrayTypeHandler} t ON u.id = t.id
</select>
```
其中 `${ids}` 表示数组入参,`typeHandler` 属性指定了使用哪个自定义类型处理器将数组转换为临时表。在 SQL 语句中,我们使用了该临时表进行 join 操作。
希望这些代码可以帮助你理解如何在 MyBatis 中将数组入参转换为临时表,并进行 join 操作。
阅读全文
相关推荐
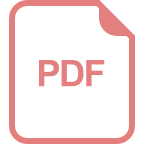
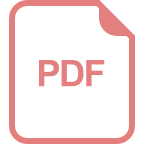
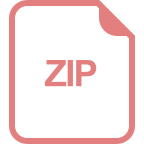















