在创建窗口句柄之前,不能在控件上调用invoke。跨窗口调用。代码实现
时间: 2024-02-28 12:53:50 浏览: 100
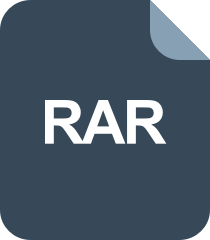
ocx.rar_C#调用ocx_OCX 控件_ocx_ocx窗口
对于跨窗口调用,可以使用委托(Delegate)的方式实现。以下是一个简单的示例代码:
```C#
// 定义一个委托类型
delegate void InvokeDelegate();
// 在主窗口中创建一个控件
public partial class MainForm : Form
{
private Button button1;
public MainForm()
{
InitializeComponent();
// 创建一个按钮控件
button1 = new Button();
button1.Text = "Click me";
button1.Location = new Point(50, 50);
button1.Click += new EventHandler(button1_Click);
this.Controls.Add(button1);
}
// 按钮点击事件处理函数
private void button1_Click(object sender, EventArgs e)
{
// 创建一个子窗口
SubForm subForm = new SubForm();
// 定义委托
InvokeDelegate invokeDelegate = new InvokeDelegate(subForm.UpdateLabel);
// 跨窗口调用
subForm.Invoke(invokeDelegate);
}
}
// 子窗口
public partial class SubForm : Form
{
private Label label1;
public SubForm()
{
InitializeComponent();
// 创建一个标签控件
label1 = new Label();
label1.Text = "Hello, world!";
label1.Location = new Point(50, 50);
this.Controls.Add(label1);
}
// 更新标签内容
public void UpdateLabel()
{
label1.Text = "Button clicked!";
}
}
```
在这个示例代码中,当用户点击主窗口中的按钮时,会创建一个子窗口,并且在子窗口中更新一个标签控件的内容。由于更新标签控件的操作需要在子窗口的线程中执行,因此我们使用了委托的方式实现跨窗口调用。在委托中调用子窗口中的方法,然后使用 Invoke() 方法将委托在子窗口的线程中执行。这样就可以避免在控件上直接调用 Invoke() 方法导致的异常。
阅读全文
相关推荐
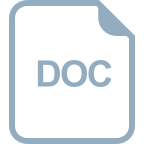
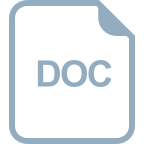


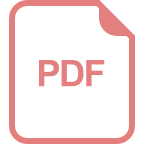
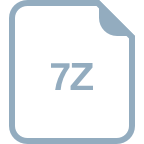
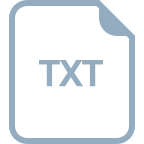
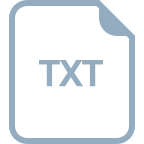
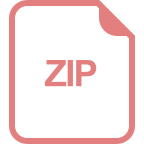
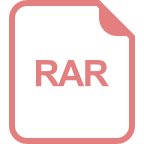
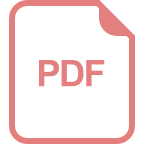
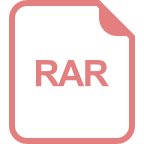
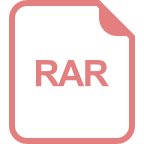
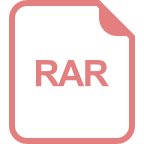
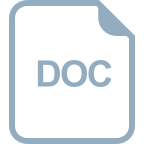
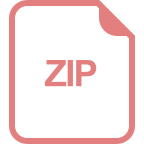

