如何在鸿蒙开发中配置请求http接口
时间: 2024-04-30 08:18:10 浏览: 152
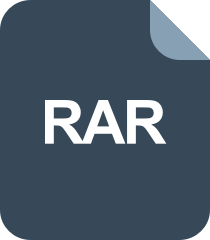
【鸿蒙版axios请求封装包】
在鸿蒙开发中,可以使用HttpURLConnection或者OkHttp来发送HTTP请求。
其中,HttpURLConnection是Android提供的网络请求框架,可以在鸿蒙上使用。具体配置步骤如下:
1. 在build.gradle文件中添加以下依赖:
```
dependencies {
implementation 'com.squareup.okhttp3:okhttp:4.9.0'
}
```
2. 在代码中使用HttpURLConnection发送HTTP请求:
```
import java.net.HttpURLConnection;
import java.net.URL;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class HttpUtil {
public static String sendHttpRequest(String urlStr) {
StringBuilder result = new StringBuilder();
try {
URL url = new URL(urlStr);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000);
connection.setReadTimeout(5000);
connection.connect();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
reader.close();
connection.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
return result.toString();
}
}
```
其中,sendHttpRequest方法中的urlStr参数为请求的URL地址,调用该方法即可发送HTTP请求。
如果想要使用OkHttp发送HTTP请求,可以参考以下示例代码:
```
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class HttpUtil {
public static String sendHttpRequest(String urlStr) {
String result = "";
try {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(urlStr)
.build();
Response response = client.newCall(request).execute();
result = response.body().string();
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
```
使用OkHttp发送HTTP请求的步骤与使用HttpURLConnection类似,只需要在代码中进行相应的调整即可。
阅读全文
相关推荐
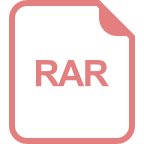
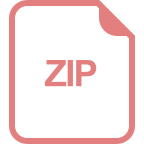















