Python中os.getcwd()的实例用法
时间: 2023-05-19 08:04:10 浏览: 80
os.getcwd()函数用于获取当前工作目录,即当前Python脚本工作的目录路径。下面是一个示例用法:
import os
# 获取当前工作目录
current_dir = os.getcwd()
print("当前工作目录为:", current_dir)
输出结果为:
当前工作目录为: /Users/username/Documents
注意:这里的路径是示例路径,实际路径可能会不同。
阅读全文
相关推荐
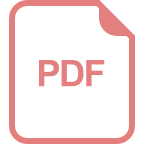
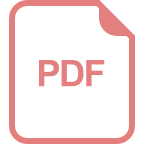
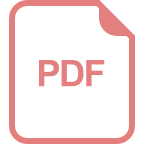
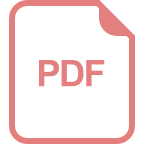
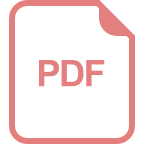
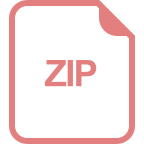
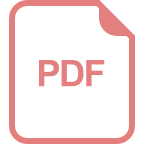
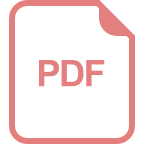
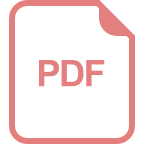
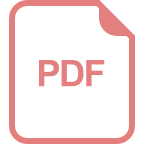
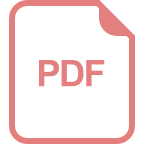
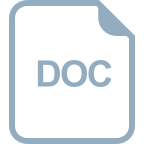
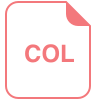
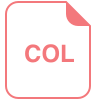
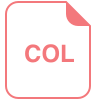
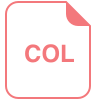
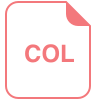
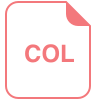
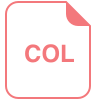