用C语言写一个SURF特征匹配程序,不依赖第三方库
时间: 2023-09-12 22:13:40 浏览: 122
好的,我来为您解答。
SURF(Speeded Up Robust Features)是一种基于尺度不变特征变换(Scale-Invariant Feature Transform,SIFT)算法的改进,它可以在不同的尺度和旋转下对图像进行特征提取和匹配。
以下是一个用C语言实现SURF特征匹配程序的基本步骤:
1. 读取输入图像,并将其转换为灰度图像。
2. 对灰度图像进行尺度空间构建,生成一组高斯差分金字塔,然后在每个图像中检测局部极值点。
3. 对每个检测到的局部极值点,生成尺度不变描述符。
4. 对两幅图像中的描述符进行匹配,计算它们之间的距离,并找到最近邻的描述符。
5. 根据最近邻描述符之间的距离,确定它们是否匹配。
下面是一个基本的SURF特征匹配程序的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 1000
#define MAX_DIM 128
typedef struct {
float x, y;
} point_t;
typedef struct {
float desc[MAX_DIM];
} feature_t;
typedef struct {
point_t point;
feature_t feature;
} keypoint_t;
int num_points1, num_points2;
keypoint_t keypoints1[MAX_POINTS], keypoints2[MAX_POINTS];
void load_image(const char *filename, int *width, int *height, unsigned char **data)
{
// Load image data from file
}
void detect_keypoints(unsigned char *image_data, int width, int height, keypoint_t *keypoints, int *num_points)
{
// Detect keypoints using SURF algorithm
}
void compute_descriptors(unsigned char *image_data, int width, int height, keypoint_t *keypoints, int num_points)
{
// Compute descriptors for keypoints using SURF algorithm
}
int match_descriptors(keypoint_t *keypoints1, int num_points1, keypoint_t *keypoints2, int num_points2, int *matches)
{
// Match descriptors between two sets of keypoints
}
int main(int argc, char **argv)
{
unsigned char *image_data1, *image_data2;
int width1, height1, width2, height2;
// Load input images
load_image(argv[1], &width1, &height1, &image_data1);
load_image(argv[2], &width2, &height2, &image_data2);
// Detect keypoints and compute descriptors for both images
detect_keypoints(image_data1, width1, height1, keypoints1, &num_points1);
compute_descriptors(image_data1, width1, height1, keypoints1, num_points1);
detect_keypoints(image_data2, width2, height2, keypoints2, &num_points2);
compute_descriptors(image_data2, width2, height2, keypoints2, num_points2);
// Match descriptors between the two sets of keypoints
int num_matches = match_descriptors(keypoints1, num_points1, keypoints2, num_points2, matches);
// Print out the number of matches found
printf("Found %d matches\n", num_matches);
// Cleanup
free(image_data1);
free(image_data2);
return 0;
}
```
注意,这只是一个简单的示例代码,实际上SURF特征匹配算法还有很多细节需要处理,比如如何选择关键点、如何计算特征描述符等。如果您想要实现更加完整和高效的SURF特征匹配程序,建议参考相关的研究论文或者开源代码。
阅读全文
相关推荐
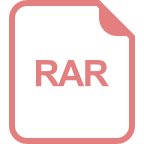
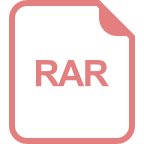
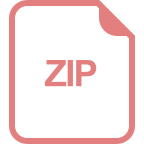
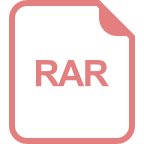
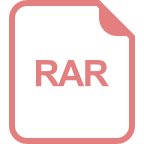
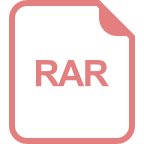
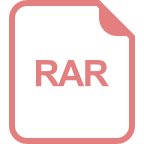
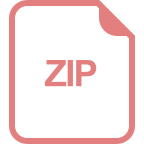
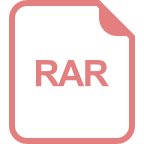
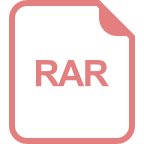
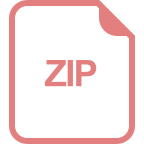
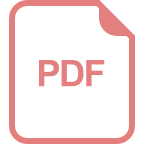
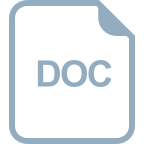


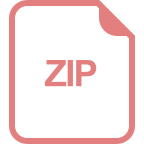