python 正则表达式如何使用
时间: 2023-10-27 16:20:57 浏览: 152
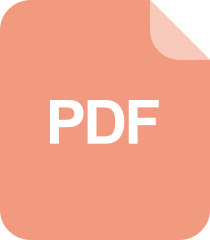
Python正则表达式使用经典实例

在 Python 中,可以使用 `re` 模块来操作正则表达式。下面是一个简单的例子,演示如何使用正则表达式来匹配一个字符串中的数字:
```python
import re
# 定义一个字符串
text = "My phone number is 123-456-7890"
# 使用正则表达式匹配数字
pattern = r'\d+' # 匹配一个或多个数字
match = re.search(pattern, text)
# 输出匹配结果
if match:
print(match.group())
else:
print("No match")
```
在上面的代码中,我们使用 `re.search()` 函数来匹配字符串中的数字。`r'\d+'` 表示一个或多个数字,`\d` 是一个特殊字符,表示数字。`match.group()` 返回匹配的字符串,如果没有匹配到则返回 `None`。
除了 `re.search()` 函数以外,还有很多其他的函数可以使用,例如 `re.findall()`、`re.sub()` 等等。要学习更多关于正则表达式在 Python 中的使用,可以查看 Python 官方文档中关于 `re` 模块的介绍。
阅读全文
相关推荐
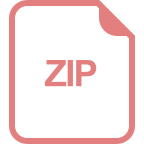
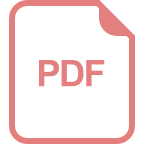















