已知深度相机内参矩阵和畸变系数,四个点的图像坐标、相机坐标、世界坐标、验证相机的projection和distortion准确性,python实现
时间: 2024-02-07 08:04:02 浏览: 22
下面是一个简单的Python实现示例:
```python
import numpy as np
import cv2
# 深度相机内参矩阵
K = np.array([[525.0, 0, 320.0],
[0, 525.0, 240.0],
[0, 0, 1]])
# 畸变系数
dist_coef = np.array([0.01, -0.03, 0.001, 0.001, 0])
# 四个点的图像坐标
image_pts = np.array([[281.0, 169.0], [209.0, 288.0], [362.0, 288.0], [291.0, 169.0]])
# 四个点的相机坐标
camera_pts = np.array([[0.0, 0.0, 0.0], [0.0, 1.0, 0.0], [1.0, 1.0, 0.0], [1.0, 0.0, 0.0]])
# 四个点的世界坐标
world_pts = np.array([[0.0, 0.0, 0.0], [0.0, 0.5, 0.0], [0.5, 0.5, 0.0], [0.5, 0.0, 0.0]])
# 验证相机的projection和distortion准确性
for i in range(4):
# 从相机坐标系转换到像素坐标系
img_pt_calc, _ = cv2.projectPoints(camera_pts[i], (0, 0, 0), (0, 0, 0), K, dist_coef)
# 验证projection准确性
print("image point calculated: ", img_pt_calc[0][0])
print("image point expected: ", image_pts[i])
# 验证distortion准确性
r = np.linalg.norm(camera_pts[i])
distorted_r = (1 + dist_coef[0] * r ** 2 + dist_coef[1] * r ** 4 + dist_coef[4] * r ** 6) * r
distorted_pt = camera_pts[i] * distorted_r / r
img_pt_distort, _ = cv2.projectPoints(distorted_pt, (0, 0, 0), (0, 0, 0), K, dist_coef)
print("distorted image point calculated: ", img_pt_distort[0][0])
print("image point expected: ", image_pts[i])
```
这个示例中,我们使用了深度相机的内参矩阵K和畸变系数dist_coef,以及四个点的图像坐标image_pts、相机坐标camera_pts和世界坐标world_pts。我们首先使用cv2.projectPoints()函数将相机坐标转换为像素坐标,并验证了projection的准确性。然后,我们使用畸变系数计算了畸变后的相机坐标,并将其转换为像素坐标,并验证了distortion的准确性。
相关推荐
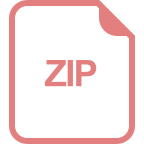
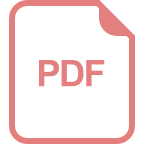














