线性表子系统代码
时间: 2023-07-03 15:26:28 浏览: 84
以下是一个简单的线性表子系统的代码示例,包含了常见的操作函数:
```c++
#include <iostream>
using namespace std;
const int MAX_SIZE = 100; // 线性表的最大容量
template<typename T>
class LinearList {
private:
T data[MAX_SIZE]; // 存储线性表的数组
int length; // 线性表的当前长度
public:
LinearList() {
length = 0;
}
// 获取线性表的长度
int getLength() {
return length;
}
// 根据下标获取线性表中的元素
T get(int index) {
if (index < 0 || index >= length) {
cout << "Index out of range." << endl;
exit(1);
}
return data[index];
}
// 在线性表的指定位置插入元素
void insert(int index, T value) {
if (index < 0 || index > length) {
cout << "Index out of range." << endl;
exit(1);
}
if (length == MAX_SIZE) {
cout << "The list is full." << endl;
exit(1);
}
for (int i = length - 1; i >= index; i--) {
data[i + 1] = data[i];
}
data[index] = value;
length++;
}
// 删除线性表中指定位置的元素
void remove(int index) {
if (index < 0 || index >= length) {
cout << "Index out of range." << endl;
exit(1);
}
for (int i = index + 1; i < length; i++) {
data[i - 1] = data[i];
}
length--;
}
// 在线性表末尾添加元素
void append(T value) {
if (length == MAX_SIZE) {
cout << "The list is full." << endl;
exit(1);
}
data[length] = value;
length++;
}
// 清空线性表
void clear() {
length = 0;
}
// 打印线性表中的元素
void print() {
for (int i = 0; i < length; i++) {
cout << data[i] << " ";
}
cout << endl;
}
};
```
通过这个线性表子系统,你可以创建一个支持插入、删除、获取、打印等操作的线性表对象,并在程序中使用它来进行数据处理。
相关推荐
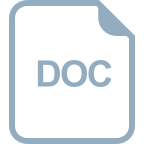
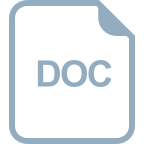














