c++ 注入DLL 代码
时间: 2023-05-27 07:03:09 浏览: 186
以下是C语言代码实现DLL注入:
```c
#include <Windows.h>
#include <TlHelp32.h>
#include <iostream>
using namespace std;
DWORD GetProcessID(LPCTSTR processName) {
PROCESSENTRY32 pe32;
HANDLE hProcessSnap;
DWORD dwProcessId = 0;
hProcessSnap = CreateToolhelp32Snapshot(TH32CS_SNAPPROCESS, 0);
if (hProcessSnap == INVALID_HANDLE_VALUE) {
cout << "CreateToolhelp32Snapshot failed.\n";
return 0;
}
pe32.dwSize = sizeof(PROCESSENTRY32);
if (!Process32First(hProcessSnap, &pe32)) {
cout << "Process32First failed.\n";
CloseHandle(hProcessSnap);
return 0;
}
do {
if (_tcscmp(pe32.szExeFile, processName) == 0) {
dwProcessId = pe32.th32ProcessID;
break;
}
} while (Process32Next(hProcessSnap, &pe32));
CloseHandle(hProcessSnap);
if (dwProcessId == 0) {
cout << "Cannot find the process: " << processName << endl;
}
return dwProcessId;
}
int main(int argc, char* argv[]) {
TCHAR dllPath[MAX_PATH] = TEXT("DLL_PATH"); // DLL文件路径
LPCTSTR processName = TEXT("PROCESS_NAME"); // 目标进程名称
DWORD dwProcessId = GetProcessID(processName);
if (dwProcessId != 0) {
HANDLE hProcess = OpenProcess(PROCESS_ALL_ACCESS, FALSE, dwProcessId);
if (hProcess == NULL) {
cout << "OpenProcess failed.\n";
return 1;
}
LPVOID pRemoteBuf = VirtualAllocEx(hProcess, NULL, MAX_PATH, MEM_COMMIT, PAGE_READWRITE);
if (pRemoteBuf == NULL) {
cout << "VirtualAllocEx failed.\n";
CloseHandle(hProcess);
return 1;
}
if (!WriteProcessMemory(hProcess, pRemoteBuf, (LPVOID)dllPath, MAX_PATH, NULL)) {
cout << "WriteProcessMemory failed.\n";
VirtualFreeEx(hProcess, pRemoteBuf, 0, MEM_RELEASE);
CloseHandle(hProcess);
return 1;
}
LPTHREAD_START_ROUTINE pThreadProc = (LPTHREAD_START_ROUTINE)GetProcAddress(GetModuleHandle(TEXT("kernel32.dll")), "LoadLibraryW");
HANDLE hThread = CreateRemoteThread(hProcess, NULL, 0, pThreadProc, pRemoteBuf, 0, NULL);
if (hThread == NULL) {
cout << "CreateRemoteThread failed.\n";
VirtualFreeEx(hProcess, pRemoteBuf, 0, MEM_RELEASE);
CloseHandle(hProcess);
return 1;
}
WaitForSingleObject(hThread, INFINITE);
VirtualFreeEx(hProcess, pRemoteBuf, 0, MEM_RELEASE);
CloseHandle(hThread);
CloseHandle(hProcess);
}
return 0;
}
```
代码中需要填写DLL文件路径和目标进程名称,然后通过调用GetProcessID函数获取指定进程的进程ID,最后将DLL路径写入目标进程并在其中注入代码。需要注意的是,在64位系统下编译和运行时需要开启x64平台支持。
阅读全文
相关推荐
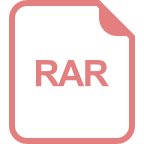
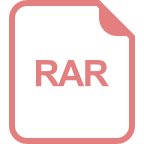
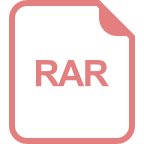
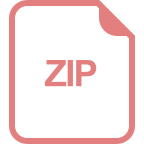
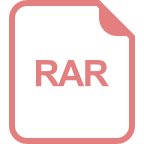
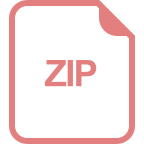
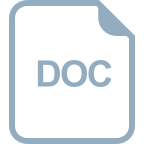
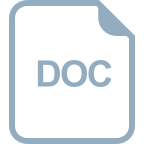
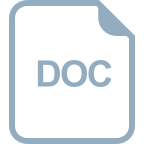
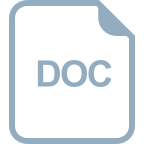
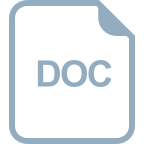
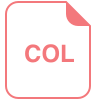



